用uci文件的api读取list用C语言实现
时间: 2024-05-16 15:14:25 浏览: 17
读取UCI文件的API可以使用以下步骤:
1. 打开文件并读取内容。
2. 逐行读取文件内容并将其存储在字符串数组中。
3. 遍历字符串数组并解析每个属性及其值。
4. 将属性及其值存储在适当的数据结构中,例如链表或哈希表。
5. 关闭文件并返回数据结构。
以下是一个简单的示例代码,用于读取名为input.uci的UCI文件中的数据并将其存储在链表中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 256
typedef struct uci_property {
char *name;
char *value;
struct uci_property *next;
} uci_property_t;
uci_property_t *uci_read_file(char *filename) {
FILE *fp;
char line[MAX_LINE_LEN];
char *name, *value;
uci_property_t *head = NULL, *tail = NULL, *prop;
// Open file for reading
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return NULL;
}
// Loop over lines in file
while (fgets(line, MAX_LINE_LEN, fp) != NULL) {
// Remove trailing newline character
line[strcspn(line, "\n")] = '\0';
// Split line into name and value
name = strtok(line, " ");
value = strtok(NULL, " ");
// Allocate new property
prop = (uci_property_t *)malloc(sizeof(uci_property_t));
prop->name = strdup(name);
prop->value = strdup(value);
prop->next = NULL;
// Add property to linked list
if (tail == NULL) {
head = tail = prop;
} else {
tail->next = prop;
tail = prop;
}
}
// Close file
fclose(fp);
return head;
}
int main() {
uci_property_t *props, *prop;
// Read UCI file
props = uci_read_file("input.uci");
// Print properties
for (prop = props; prop != NULL; prop = prop->next) {
printf("%s = %s\n", prop->name, prop->value);
}
// Free memory
for (prop = props; prop != NULL; prop = prop->next) {
free(prop->name);
free(prop->value);
free(prop);
}
return 0;
}
```
该例程使用fgets()函数逐行读取文件内容,并使用strtok()函数将每一行拆分成属性名和属性值。然后,它将每个属性及其值存储在一个链表中,并在读取完整个文件后返回该链表。最后,它遍历链表并打印每个属性及其值,并释放链表中的所有内存。
请注意,该示例代码没有进行错误检查,例如检查属性名和属性值是否为NULL。在实际应用中,您应该添加适当的错误检查来确保代码的稳健性。
相关推荐
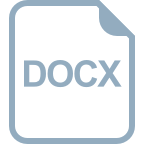














