定义一个int型的数组,包含10个元素,分别赋值为0~9,然后将数组中的元素都往前移一个位置, 即:a[0]=a[1],a[1]=a[2],...最后一个元素的值是原来第一个元素的值,然后输出这个数组, 演示格式如下:1 2 3 4 5 6 7 8 9 0
时间: 2023-05-30 16:04:34 浏览: 161
代码如下:
```java
public class Main {
public static void main(String[] args) {
int[] a = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
int temp = a[0];
for (int i = 1; i < a.length; i++) {
a[i - 1] = a[i];
}
a[a.length - 1] = temp;
for (int i = 0; i < a.length; i++) {
System.out.print(a[i] + " ");
}
}
}
```
输出结果为:1 2 3 4 5 6 7 8 9 0
相关问题
5.给定一个整数数组 nums,将所有 0 移动到数组的末尾,同时保持非零元素的相对顺序。
这道题可以使用双指针法,将非零元素前移,将0后移,直到所有0都移到了数组的末尾。
具体实现:
1. 定义两个指针i和j,初始值都为0。
2. 遍历数组,如果nums[i]不等于0,则将其赋值给nums[j],并将i和j均加1;否则,只将i加1。
3. 遍历结束后,从j开始到数组的末尾,将剩余的元素赋值为0。
代码如下:
```
void moveZeroes(vector<int>& nums) {
int i = 0, j = 0;
while (i < nums.size()) {
if (nums[i] != 0) {
nums[j] = nums[i];
++j;
}
++i;
}
while (j < nums.size()) {
nums[j] = 0;
++j;
}
}
```
时间复杂度为O(n),空间复杂度为O(1)。
java数组整体前移
以下是Java数组整体前移的示例代码:
```java
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
System.out.println("移动前:" + Arrays.toString(arr));
int temp = arr[0];
for (int i = 1; i < arr.length; i++) {
arr[i - 1] = arr[i];
}
arr[arr.length - 1] = temp;
System.out.println("移动后:" + Arrays.toString(arr));
}
```
上述代码中,我们首先定义了一个整型数组 `arr`,然后使用 `Arrays.toString()` 方法打印出数组元素。接着,我们将数组的第一个元素保存到临时变量 `temp` 中,然后使用循环将数组中的元素整体前移一位。最后,将 `temp` 赋值给数组的最后一个元素,完成整体前移操作。
阅读全文
相关推荐
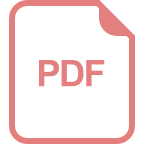
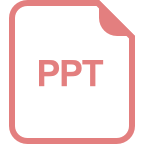
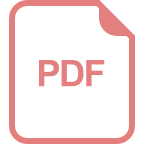













