在Django Rest Framework中,有一个comment评论模型中有content(TextField)字段、student(ForeignKey)字段。有一个Reply回复模型中有content(TextField)字段、comments(ForeignKey)字段。还有一个Notification通知模型中content(TextField)字段、student(ForeignKey)字段,现在需要一个通知功能,该功能为:每当有一个comment或是reply生成,就向student发送一个通知。
时间: 2024-03-11 15:47:33 浏览: 10
对于这个需求,你可以使用Django signals来实现。Signals是Django提供的一个事件机制,可以在模型的save、delete、update等操作中注册回调函数,在这些操作被执行时自动调用回调函数。
首先,你需要在models.py中定义Comment,Reply和Notification模型,并在每个模型的save方法中添加信号,如下所示:
```python
from django.db import models
from django.dispatch import receiver
from django.db.models.signals import post_save
class Student(models.Model):
name = models.CharField(max_length=100)
class Comment(models.Model):
content = models.TextField()
student = models.ForeignKey(Student, on_delete=models.CASCADE)
@receiver(post_save, sender='yourapp.Comment')
def create_notification(sender, instance, created, **kwargs):
if created:
Notification.objects.create(
content=f'New comment from {instance.student.name}',
student=instance.student
)
class Reply(models.Model):
content = models.TextField()
comment = models.ForeignKey(Comment, on_delete=models.CASCADE)
@receiver(post_save, sender='yourapp.Reply')
def create_notification(sender, instance, created, **kwargs):
if created:
Notification.objects.create(
content=f'New reply to comment from {instance.comment.student.name}',
student=instance.comment.student
)
class Notification(models.Model):
content = models.TextField()
student = models.ForeignKey(Student, on_delete=models.CASCADE)
```
在上面的代码中,我们在Comment和Reply模型中定义了 create_notification 函数,并使用 @receiver 装饰器将其注册为 post_save 信号的回调函数。当 Comment 或 Reply 模型的实例被保存时,create_notification 函数会被自动调用。在该函数中,我们创建了一个新的 Notification 实例,并将其保存到数据库中。
注意,我们在 create_notification 函数中使用了 created 参数来判断该实例是新创建的还是更新了已有的实例。这样做可以避免在更新实例时重复创建通知。
最后,你需要在你想要发送通知的地方调用 Comment 或 Reply 模型的 save 方法,就可以自动触发信号并创建通知了。
相关推荐
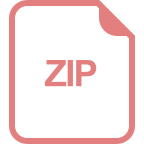
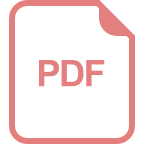
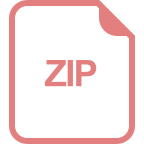














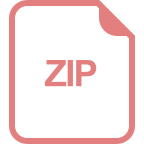