qml实现数字文本输入
时间: 2024-05-07 13:21:20 浏览: 12
可以使用 Qt Quick Controls 里的 TextInput 组件实现数字文本输入,具体步骤如下:
1. 在 QML 文件中导入 Qt Quick 和 Qt Quick Controls:
```
import QtQuick 2.15
import QtQuick.Controls 2.15
```
2. 使用 TextInput 组件创建一个文本输入框,并设置其 inputMask 为数字格式:
```
TextInput {
id: numberInput
inputMask: "D"
}
```
其中,inputMask 属性的值 "D" 表示只允许输入数字,如果需要限制输入数字的位数,可以使用类似 "D99" 的格式。
3. 可以通过绑定 text 属性来获取用户输入的数字值:
```
property int inputValue: 0
TextInput {
id: numberInput
inputMask: "D"
onTextChanged: {
if (text !== "") {
inputValue = parseInt(text)
}
}
}
```
在这个例子中,我们定义了一个名为 inputValue 的属性,用于存储用户输入的数字值。当用户在文本输入框中输入数字时,我们将其转换为整数并赋值给 inputValue。
4. 可以使用 validator 属性来添加额外的输入验证,例如限制输入范围:
```
TextInput {
id: numberInput
inputMask: "D"
validator: IntValidator {
bottom: 0
top: 100
}
}
```
在这个例子中,我们使用 IntValidator 来限制用户输入的数字必须在 0 到 100 之间。
完整的代码示例:
```
import QtQuick 2.15
import QtQuick.Controls 2.15
Item {
width: 200
height: 200
property int inputValue: 0
TextInput {
id: numberInput
inputMask: "D"
validator: IntValidator {
bottom: 0
top: 100
}
onTextChanged: {
if (text !== "") {
inputValue = parseInt(text)
}
}
}
Text {
text: "Input value: " + inputValue
anchors.top: numberInput.bottom
anchors.horizontalCenter: numberInput.horizontalCenter
}
}
```
相关推荐
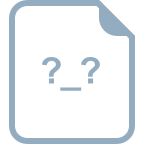
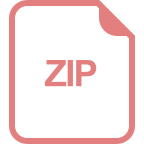














