python 判断图片是否重名
时间: 2023-07-08 18:47:33 浏览: 93
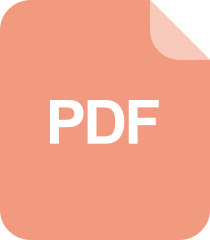
python实现大量图片重命名
可以使用Python的os模块和glob模块来实现判断图片是否重名的功能。
首先,可以使用os模块的listdir函数来获取指定目录下的所有文件名,然后使用glob模块的glob函数来筛选出所有图片文件的文件名,如下所示:
```python
import os
import glob
dir_path = '/path/to/directory' # 指定目录路径
img_files = glob.glob(os.path.join(dir_path, '*.jpg')) # 筛选出所有以.jpg结尾的文件名
```
接着,可以使用Python的集合(Set)来判断图片文件名是否重复,如下所示:
```python
if len(img_files) == len(set(img_files)):
print('图片文件名没有重名')
else:
print('图片文件名存在重名')
```
其中,集合的长度和列表的长度相同时,说明没有重复元素,否则说明存在重复元素。
需要注意的是,上述代码只是通过文件名来判断是否重名,如果有多个不同目录下的文件名相同,也会被判断为重名。如果需要更精确的判断,可以考虑使用文件的MD5值或者SHA1值等来判断文件是否相同。
阅读全文
相关推荐
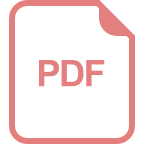
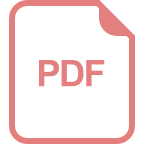

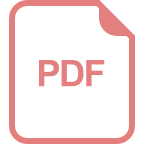
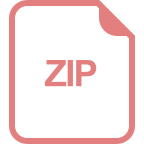

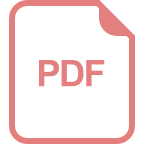
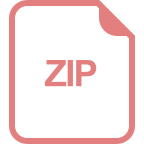
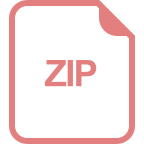
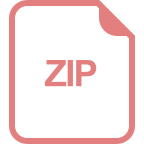
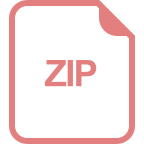
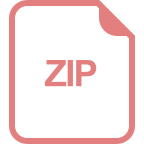
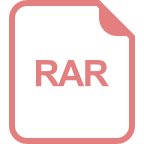
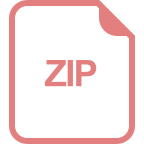
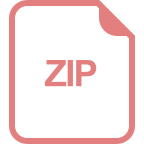