生成一段c语言五子棋判断胜负的代码
时间: 2023-12-08 14:06:37 浏览: 87
以下是一段简单的 C 语言五子棋判断胜负的代码:
```c
#include <stdio.h>
#define SIZE 15
int board[SIZE][SIZE]; // 0 表示空,1 表示黑棋,2 表示白棋
int checkWin(int x, int y, int player) {
int i, j, count;
// 检查行
count = 1;
for (i = x + 1; i < SIZE && board[i][y] == player; i++) count++;
for (i = x - 1; i >= 0 && board[i][y] == player; i--) count++;
if (count >= 5) return 1;
// 检查列
count = 1;
for (j = y + 1; j < SIZE && board[x][j] == player; j++) count++;
for (j = y - 1; j >= 0 && board[x][j] == player; j--) count++;
if (count >= 5) return 1;
// 检查正对角线
count = 1;
for (i = x + 1, j = y + 1; i < SIZE && j < SIZE && board[i][j] == player; i++, j++) count++;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == player; i--, j--) count++;
if (count >= 5) return 1;
// 检查反对角线
count = 1;
for (i = x + 1, j = y - 1; i < SIZE && j >= 0 && board[i][j] == player; i++, j--) count++;
for (i = x - 1, j = y + 1; i >= 0 && j < SIZE && board[i][j] == player; i--, j++) count++;
if (count >= 5) return 1;
return 0;
}
int main() {
int x, y, player = 1;
while (1) {
printf("请输入坐标(x, y):");
scanf("%d%d", &x, &y);
if (x < 0 || x >= SIZE || y < 0 || y >= SIZE) {
printf("坐标不合法,请重新输入!\n");
continue;
}
if (board[x][y] != 0) {
printf("该位置已经有棋子,请重新输入!\n");
continue;
}
board[x][y] = player;
if (checkWin(x, y, player)) {
printf("恭喜玩家 %d 获胜!\n", player);
break;
}
player = 3 - player;
}
return 0;
}
```
该代码实现了一个简单的五子棋游戏,玩家可以输入坐标来落子,程序会判断胜负并输出结果。其中 `checkWin` 函数用于判断当前落子是否导致获胜,它会检查当前位置所在的行、列、正对角线和反对角线是否有五个连续的同色棋子。
阅读全文
相关推荐



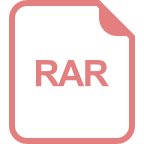










