hi,我现在想写一个pytorch模型,首先将输入的特征通过autoencoder得到压缩的特征,然后把压缩后的特征concat到原来的输入上,然后再把concat得到的输入通过一个神经网络。请问你能给我一段代码示例,并同时给我一段文字描述吗?
时间: 2024-02-17 21:02:00 浏览: 68
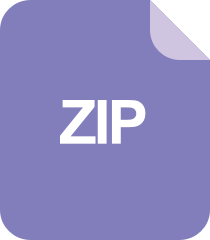
基于pytorch+python实现一个中文到英文的神经网络的翻译模型.zip

当然可以。首先,我们需要定义一个自编码器模型,它将输入的特征压缩为一个较小的向量。以下是一个简单的自编码器模型的示例代码:
```python
import torch
import torch.nn as nn
class AutoEncoder(nn.Module):
def __init__(self):
super(AutoEncoder, self).__init__()
self.encoder = nn.Sequential(
nn.Linear(784, 128),
nn.ReLU(),
nn.Linear(128, 64),
nn.ReLU(),
nn.Linear(64, 12),
nn.ReLU(),
nn.Linear(12, 2))
self.decoder = nn.Sequential(
nn.Linear(2, 12),
nn.ReLU(),
nn.Linear(12, 64),
nn.ReLU(),
nn.Linear(64, 128),
nn.ReLU(),
nn.Linear(128, 784),
nn.Sigmoid())
def forward(self, x):
x = self.encoder(x)
x = self.decoder(x)
return x
```
这个自编码器模型将输入的特征向量压缩为一个长度为2的向量。接下来,我们可以定义一个神经网络模型,它将原始特征和压缩特征连接起来,并将它们传递给一个完全连接的层:
```python
class ConcatNet(nn.Module):
def __init__(self):
super(ConcatNet, self).__init__()
self.autoencoder = AutoEncoder()
self.fc = nn.Linear(794, 10)
def forward(self, x):
compressed = self.autoencoder.encoder(x)
x = torch.cat([x, compressed], dim=1)
x = self.fc(x)
return x
```
在这个模型中,我们首先通过自编码器模型将输入的特征压缩为一个长度为2的向量。然后,我们使用`torch.cat`函数将原始特征和压缩特征连接起来。最后,我们将连接的特征传递给一个完全连接的层。
希望这可以帮助到你。
阅读全文
相关推荐
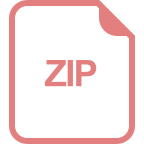
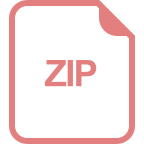

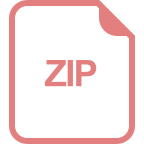
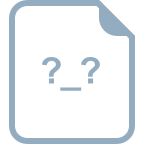
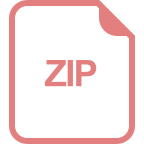
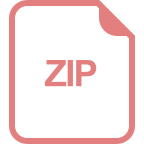
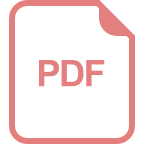
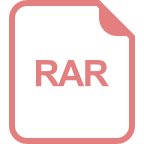
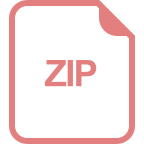
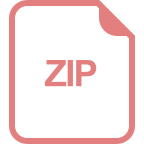
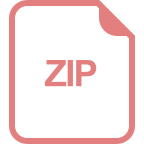
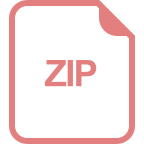