Java连接数据库写一个产品销售项目
时间: 2023-06-17 13:08:08 浏览: 180
好的,以下是一个简单的Java连接数据库的产品销售项目的示例:
1. 创建一个名为Product的Java类,表示产品实体,包括属性id、name、description、price和quantity。
```
public class Product {
private int id;
private String name;
private String description;
private double price;
private int quantity;
public Product(int id, String name, String description, double price, int quantity) {
this.id = id;
this.name = name;
this.description = description;
this.price = price;
this.quantity = quantity;
}
// getter and setter methods
}
```
2. 创建一个名为ProductDAO的Java类,用于执行与数据库交互的操作,包括连接到数据库、插入产品、更新产品、删除产品和获取所有产品等操作。
```
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class ProductDAO {
private String jdbcURL;
private String jdbcUsername;
private String jdbcPassword;
private Connection jdbcConnection;
public ProductDAO(String jdbcURL, String jdbcUsername, String jdbcPassword) {
this.jdbcURL = jdbcURL;
this.jdbcUsername = jdbcUsername;
this.jdbcPassword = jdbcPassword;
}
//连接数据库
protected void connect() throws SQLException {
if (jdbcConnection == null || jdbcConnection.isClosed()) {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
} catch (ClassNotFoundException e) {
throw new SQLException(e);
}
jdbcConnection = DriverManager.getConnection(
jdbcURL, jdbcUsername, jdbcPassword);
}
}
//断开数据库连接
protected void disconnect() throws SQLException {
if (jdbcConnection != null && !jdbcConnection.isClosed()) {
jdbcConnection.close();
}
}
//插入产品
public boolean insertProduct(Product product) throws SQLException {
String sql = "INSERT INTO product (name, description, price, quantity) VALUES (?, ?, ?, ?)";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, product.getName());
statement.setString(2, product.getDescription());
statement.setDouble(3, product.getPrice());
statement.setInt(4, product.getQuantity());
boolean rowInserted = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowInserted;
}
//更新产品
public boolean updateProduct(Product product) throws SQLException {
String sql = "UPDATE product SET name = ?, description = ?, price = ?, quantity = ? WHERE id = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, product.getName());
statement.setString(2, product.getDescription());
statement.setDouble(3, product.getPrice());
statement.setInt(4, product.getQuantity());
statement.setInt(5, product.getId());
boolean rowUpdated = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowUpdated;
}
//删除产品
public boolean deleteProduct(Product product) throws SQLException {
String sql = "DELETE FROM product WHERE id = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setInt(1, product.getId());
boolean rowDeleted = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowDeleted;
}
//获取所有产品
public List<Product> getAllProducts() throws SQLException {
List<Product> products = new ArrayList<>();
String sql = "SELECT * FROM product";
connect();
Statement statement = jdbcConnection.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
String description = resultSet.getString("description");
double price = resultSet.getDouble("price");
int quantity = resultSet.getInt("quantity");
Product product = new Product(id, name, description, price, quantity);
products.add(product);
}
resultSet.close();
statement.close();
disconnect();
return products;
}
}
```
3. 创建一个名为ProductApp的Java类,包括主方法,用于测试ProductDAO的各种操作。
```
import java.sql.SQLException;
import java.util.List;
public class ProductApp {
public static void main(String[] args) {
String jdbcURL = "jdbc:mysql://localhost:3306/productdb?useSSL=false";
String jdbcUsername = "root";
String jdbcPassword = "password";
ProductDAO productDAO = new ProductDAO(jdbcURL, jdbcUsername, jdbcPassword);
try {
//插入产品
Product newProduct = new Product(1, "iPhone 12", "A14 Bionic chip, Super Retina XDR display", 999.0, 10);
productDAO.insertProduct(newProduct);
//更新产品
Product existingProduct = new Product(1, "iPhone 12 Pro", "A14 Bionic chip, Pro camera system", 1099.0, 5);
productDAO.updateProduct(existingProduct);
//删除产品
Product deletedProduct = new Product(1, "", "", 0.0, 0);
productDAO.deleteProduct(deletedProduct);
//获取所有产品
List<Product> products = productDAO.getAllProducts();
for (Product product : products) {
System.out.println(product.getName());
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
```
这是一个简单的Java连接数据库的产品销售项目示例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
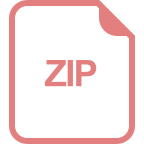
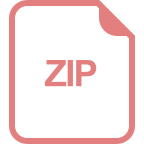
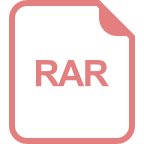
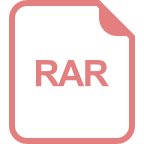
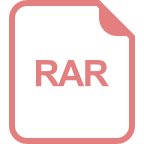
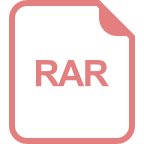
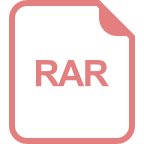
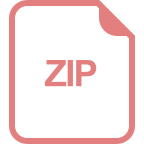
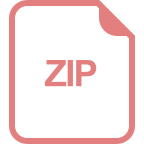
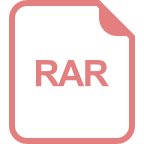
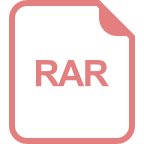
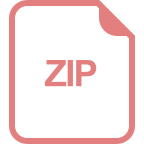
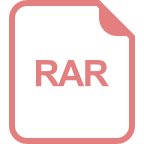
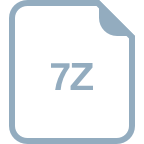
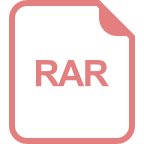
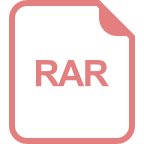