javafx的textfield设置只能输入字母和数字
时间: 2023-09-23 22:07:37 浏览: 93
您好!要实现只能输入字母和数字的TextField,可以使用JavaFX的TextFormatter类。以下是示例代码:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.control.TextFormatter;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import java.util.function.UnaryOperator;
import java.util.regex.Pattern;
public class Main extends Application {
private static final Pattern LETTERS_NUMBERS_PATTERN = Pattern.compile("[a-zA-Z0-9]*");
@Override
public void start(Stage primaryStage) throws Exception {
TextField textField = new TextField();
UnaryOperator<TextFormatter.Change> filter = change -> {
String newText = change.getControlNewText();
if (LETTERS_NUMBERS_PATTERN.matcher(newText).matches()) {
return change;
} else {
return null;
}
};
TextFormatter<String> formatter = new TextFormatter<>(filter);
textField.setTextFormatter(formatter);
StackPane root = new StackPane(textField);
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在上面的代码中,我们使用了一个正则表达式模式来限制输入只能是字母和数字。通过创建一个UnaryOperator来实现过滤器,如果输入的字符串符合模式,则返回原始的TextFormatter.Change对象,否则返回null。最后,我们将TextFormatter对象设置到TextField中。
相关推荐
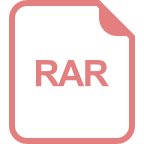














