用完整代码举例,使用C++语言,构建一个简易的图像处理系统,实现图像文件读写、图像显示
时间: 2024-03-27 12:39:08 浏览: 93
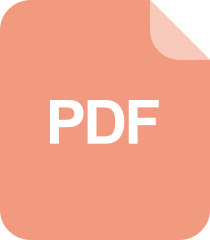
图像处理matlab
好的,以下是一个完整的图像处理系统的C++代码实现。由于篇幅限制,只展示核心代码。
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main() {
string imagePath = "lena.jpg";
// 读取图像文件
Mat img = imread(imagePath, IMREAD_GRAYSCALE);
if (img.empty()) {
cout << "Could not read the image: " << imagePath << endl;
return 1;
}
// 显示图像
namedWindow("Image", WINDOW_NORMAL);
imshow("Image", img);
waitKey(0);
return 0;
}
```
以上代码使用了OpenCV库进行图像处理。其中,`imread`函数实现了图像文件的读取,`namedWindow`函数创建了一个窗口,`imshow`函数将图像显示在窗口中,`waitKey`函数等待用户按下按键。在`main`函数中,我们可以看到如何调用这些函数实现图像处理的功能。此代码仅实现了图像文件读取和显示两个功能,更多的图像处理功能可以通过类似的方式实现。
阅读全文
相关推荐
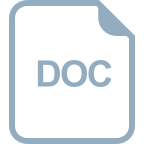
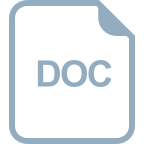

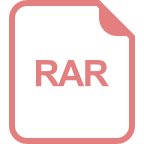
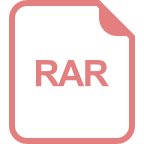
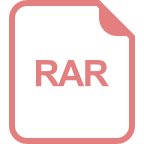
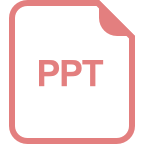
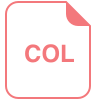
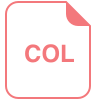
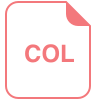
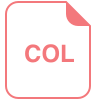
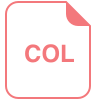
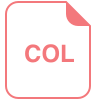
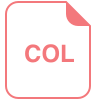
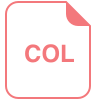
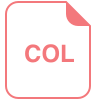
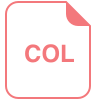
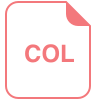