用visual studio2022写一个提取音频波形的代码
时间: 2024-05-19 11:10:48 浏览: 111
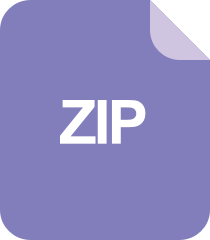
ChatGLM-6B-visualstudio2022使用教程
以下是使用 Visual Studio 2022 编写的提取音频波形的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <Windows.h>
#include <mmsystem.h>
#pragma comment(lib, "winmm.lib")
using namespace std;
int main()
{
// 打开音频文件
const char* filename = "test.wav";
ifstream file(filename, ios::binary);
if (!file)
{
cout << "File not found!" << endl;
return -1;
}
// 读取音频数据
char chunkID[4], format[4], subchunk1ID[4], subchunk2ID[4];
DWORD chunkSize, formatChunkSize, subchunk1Size, sampleRate, byteRate, subchunk2Size;
WORD audioFormat, numChannels, bitsPerSample, blockAlign;
file.read(chunkID, 4); // RIFF
file.read((char*)&chunkSize, 4); // 文件长度
file.read(format, 4); // WAVE
file.read(subchunk1ID, 4); // fmt
file.read((char*)&subchunk1Size, 4); // 格式块长度
file.read((char*)&audioFormat, 2); // 音频格式
file.read((char*)&numChannels, 2); // 声道数
file.read((char*)&sampleRate, 4); // 采样率
file.read((char*)&byteRate, 4); // 数据传输速率
file.read((char*)&blockAlign, 2); // 数据块对齐单位
file.read((char*)&bitsPerSample, 2); // 采样位数
file.read(subchunk2ID, 4); // data
file.read((char*)&subchunk2Size, 4); // 音频数据长度
vector<short> audioData(subchunk2Size / 2);
file.read((char*)audioData.data(), subchunk2Size);
// 获取音频数据最大值和最小值
short maxVal = 0, minVal = 0;
for (auto sample : audioData)
{
if (sample > maxVal)
maxVal = sample;
if (sample < minVal)
minVal = sample;
}
// 绘制音频波形
const int width = 800, height = 400;
HDC hdc = GetDC(NULL);
HPEN pen = CreatePen(PS_SOLID, 1, RGB(0, 0, 0));
SelectObject(hdc, pen);
MoveToEx(hdc, 0, height / 2, NULL);
for (int i = 0; i < audioData.size(); i++)
{
int x = i * width / audioData.size();
int y = height / 2 - (audioData[i] - minVal) * height / (maxVal - minVal);
LineTo(hdc, x, y);
}
DeleteObject(pen);
ReleaseDC(NULL, hdc);
return 0;
}
```
此代码读取 WAV 格式的音频文件,提取音频数据并绘制出音频波形。你需要将 `test.wav` 替换为你要处理的音频文件名,并将代码中的绘图部分适当修改以适应你的需求。
阅读全文
相关推荐
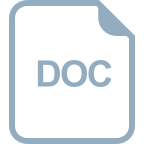
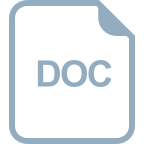

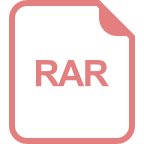
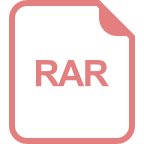
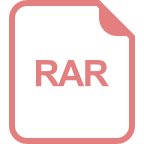
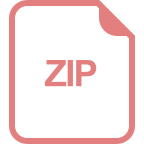
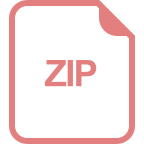
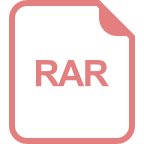
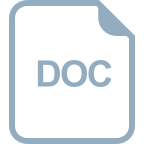
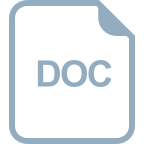
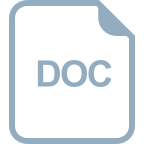
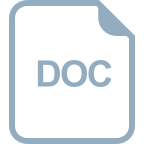
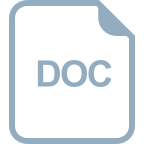
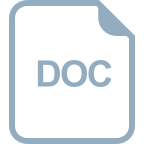
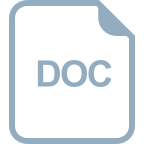
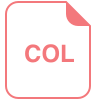
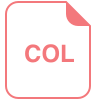