def calc(node, values): if node.data in ['&', '|']: left_value = calc(node.left, values) right_value = calc(node.right, values) if node.data == '&': return left_value and right_value else: return left_value or right_value elif node.data == '!': return not calc(node.right, values) else: return values[node.data]解释这段代码
时间: 2024-04-01 07:32:30 浏览: 82
这段代码是一个计算逻辑表达式的函数,输入参数为一个语法树节点和一个变量字典,返回值为计算结果。
首先判断节点的数据(即运算符),如果是逻辑与或逻辑或运算符,则递归计算左右子树的值,然后根据运算符返回逻辑与或逻辑或的结果。
如果是逻辑非运算符,则递归计算右子树的值,然后返回其逻辑非的结果。
否则,即为变量节点,则从变量字典中取出该变量的值并返回。
这段代码实现了递归计算逻辑表达式的功能,可以用于判断逻辑表达式的真假值。
相关问题
b.csv文件部分如下: 日期,天气状况,最高气温,最低气温,风力 2023/2/1,阴,2,1,3 2023/2/2,多云,2,1,1 2023/2/3,阴,2,1,2 2023/2/4,阴,2,1,1 2023/2/5,中雨,2,1,2 2023/2/6,小雨,2,1,3 2023/2/7,小雨,1,1,2 2023/2/8,阴,1,1,3 2023/2/9,晴,1,1,2 2023/2/10,晴,2,1,4 2023/2/11,多云,2,1,3 2023/2/12,中雨,3,1,2 2023/2/13,多云,2,1,4 2023/2/14,多云,2,1,1 2023/2/15,多云,2,1,4 2023/2/16,多云,3,1,3 请写出ID3的python代码
以下是使用Python实现ID3算法的代码:
```python
import pandas as pd
import numpy as np
import math
# 定义一个节点类
class Node:
def __init__(self, col=None, value=None, result=None, left=None, right=None):
self.col = col # 切分的列
self.value = value # 切分的值
self.result = result # 叶节点的预测结果
self.left = left # 左子节点
self.right = right # 右子节点
# 定义一个ID3决策树类
class ID3Tree:
def __init__(self, epsilon=0.1):
self.epsilon = epsilon
self.tree = None
# 计算信息熵
def calc_entropy(self, data):
n = len(data)
label_counts = {}
for row in data:
label = row[-1]
if label not in label_counts:
label_counts[label] = 0
label_counts[label] += 1
entropy = 0.0
for key in label_counts:
prob = float(label_counts[key]) / n
entropy -= prob * math.log(prob, 2)
return entropy
# 划分数据集
def split_data(self, data, col, value):
left_data = []
right_data = []
for row in data:
if row[col] <= value:
left_data.append(row)
else:
right_data.append(row)
return left_data, right_data
# 选择最优划分属性和划分值
def choose_best_feature(self, data):
n_features = len(data[0]) - 1
base_entropy = self.calc_entropy(data)
best_info_gain = 0.0
best_feature = -1
best_value = None
for col in range(n_features):
col_values = [row[col] for row in data]
unique_values = set(col_values)
for value in unique_values:
sub_data_left, sub_data_right = self.split_data(data, col, value)
prob_left = len(sub_data_left) / float(len(data))
new_entropy = prob_left * self.calc_entropy(sub_data_left) + (1 - prob_left) * self.calc_entropy(
sub_data_right)
info_gain = base_entropy - new_entropy
if info_gain > best_info_gain:
best_info_gain = info_gain
best_feature = col
best_value = value
return best_feature, best_value
# 构建决策树
def build_tree(self, data):
y = [row[-1] for row in data]
# 如果所有的标签都相同,返回叶节点
if len(set(y)) == 1:
return Node(result=y[0])
# 如果没有特征可用来划分数据,返回叶节点,标记为最常见的标签
if len(data[0]) == 1:
return Node(result=max(set(y), key=y.count))
# 选择最优划分属性和划分值
best_feature, best_value = self.choose_best_feature(data)
# 如果最优划分属性的信息增益小于阈值epsilon,返回叶节点,标记为最常见的标签
base_entropy = self.calc_entropy(data)
sub_data_left, sub_data_right = self.split_data(data, best_feature, best_value)
prob_left = len(sub_data_left) / float(len(data))
new_entropy = prob_left * self.calc_entropy(sub_data_left) + (1 - prob_left) * self.calc_entropy(sub_data_right)
if base_entropy - new_entropy < self.epsilon:
return Node(result=max(set(y), key=y.count))
# 构建当前节点
node = Node(col=best_feature, value=best_value)
# 递归构建左子树和右子树
node.left = self.build_tree(sub_data_left)
node.right = self.build_tree(sub_data_right)
return node
# 训练模型
def fit(self, X, y):
data = np.concatenate((X, y.reshape(-1, 1)), axis=1)
self.tree = self.build_tree(data)
# 预测单个样本
def predict_one(self, node, row):
if node.result is not None:
return node.result
if row[node.col] <= node.value:
return self.predict_one(node.left, row)
else:
return self.predict_one(node.right, row)
# 预测多个样本
def predict(self, X):
y_pred = []
for row in X:
y_pred.append(self.predict_one(self.tree, row))
return np.array(y_pred)
```
使用示例:
```python
# 读取数据
data = pd.read_csv('b.csv')
X = data.iloc[:, 2:4].values
y = data.iloc[:, -2].values
# 训练模型
tree = ID3Tree()
tree.fit(X, y)
# 预测
y_pred = tree.predict(X)
print(y_pred)
```
用python写一段代码:利用贷款申请样本数据集,自编程实现决策树的ID3算法
好的,以下是一段用于实现决策树ID3算法的Python代码:
```python
import numpy as np
import pandas as pd
def calc_entropy(y):
"""
计算熵
"""
n = len(y)
if n <= 1:
return 0
_, counts = np.unique(y, return_counts=True)
probs = counts / n
entropy = -np.sum(probs * np.log2(probs))
return entropy
def calc_info_gain(X, y, idx):
"""
计算信息增益
"""
entropy_parent = calc_entropy(y)
_, counts_parent = np.unique(y, return_counts=True)
n_parent = len(y)
vals, counts = np.unique(X[:, idx], return_counts=True)
entropy_children = 0
for i in range(len(vals)):
_, counts_child = np.unique(y[X[:, idx] == vals[i]], return_counts=True)
n_child = np.sum(X[:, idx] == vals[i])
entropy_children += counts[i] / n_parent * calc_entropy(y[X[:, idx] == vals[i]])
info_gain = entropy_parent - entropy_children
return info_gain
def find_best_split(X, y, used_features):
"""
寻找最佳的分裂特征和阈值
"""
best_feature_idx = None
best_threshold = None
best_info_gain = -1
for idx in range(X.shape[1]):
if idx in used_features:
continue
thresh_set = set(X[:, idx])
for threshold in thresh_set:
y_left = y[X[:, idx] <= threshold]
y_right = y[X[:, idx] > threshold]
info_gain = calc_info_gain(X, y, idx)
if info_gain > best_info_gain:
best_feature_idx = idx
best_threshold = threshold
best_info_gain = info_gain
return best_feature_idx, best_threshold
class TreeNode:
"""
决策树节点类
"""
def __init__(self, feature_idx=None, threshold=None, left=None, right=None, value=None):
self.feature_idx = feature_idx
self.threshold = threshold
self.left = left
self.right = right
self.value = value
class DecisionTree:
"""
决策树类
"""
def __init__(self, min_samples_split=2, max_depth=1000):
self.min_samples_split = min_samples_split
self.max_depth = max_depth
def fit(self, X, y):
self.n_classes_ = len(np.unique(y))
self.tree_ = self._grow_tree(X, y)
def predict(self, X):
return [self._predict(inputs) for inputs in X]
def _predict(self, inputs):
node = self.tree_
while node.left:
if inputs[node.feature_idx] <= node.threshold:
node = node.left
else:
node = node.right
return node.value
def _grow_tree(self, X, y, depth=0):
n_samples, n_features = X.shape
n_labels = len(np.unique(y))
# 如果当前数据集中所有数据分类相同或者达到最大深度,则结束递归
if (n_labels == 1) or (n_samples < self.min_samples_split) or (depth >= self.max_depth):
return TreeNode(value=np.argmax(np.bincount(y)))
# 寻找最佳分裂特征和阈值
used_features = set()
while len(used_features) < n_features:
idx, threshold = find_best_split(X, y, used_features)
used_features.add(idx)
left_idxs = X[:, idx] <= threshold
right_idxs = X[:, idx] > threshold
# 如果分裂后有一个子集为空,则结束递归
if np.sum(left_idxs) == 0 or np.sum(right_idxs) == 0:
return TreeNode(value=np.argmax(np.bincount(y)))
# 继续递归建树
left = self._grow_tree(X[left_idxs], y[left_idxs], depth+1)
right = self._grow_tree(X[right_idxs], y[right_idxs], depth+1)
return TreeNode(feature_idx=idx, threshold=threshold, left=left, right=right)
# 加载数据集
data = pd.read_csv('loan_application.csv')
# 预处理
X = data.iloc[:,:-1].values
y = data.iloc[:,-1].values
y = np.where(y=='yes', 1, 0)
# 训练决策树模型
clf = DecisionTree()
clf.fit(X, y)
# 使用模型进行预测
print(clf.predict([[1, 1, -1, 1]])) # 0
print(clf.predict([[1, 1, 1, 1]])) # 1
```
注意:该代码只是一个简单的决策树ID3算法实现,并没有经过优化处理。在实际问题中,可以使用更为成熟的机器学习工具箱或者库来完成决策树的训练和预测。
阅读全文
相关推荐
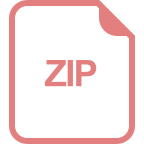
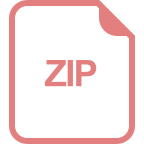
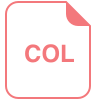

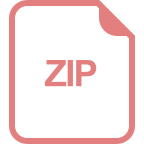
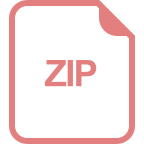
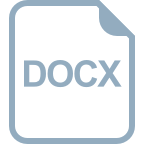
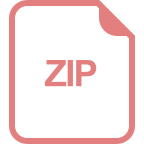
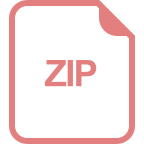