stlc++图书管理系统
时间: 2023-09-09 17:10:25 浏览: 101
STL(C++标准模板库)可以用来实现图书管理系统,其中可以使用vector来存储图书的信息,使用map来实现关键字搜索,使用algorithm来实现排序等功能。
以下是一个简单的图书管理系统的示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <map>
#include <algorithm>
using namespace std;
struct Book {
string name;
string author;
int num;
};
// 用vector存储图书信息
vector<Book> books;
// 用map存储关键字
map<string, vector<int>> index;
// 添加图书
void addBook(string name, string author, int num) {
Book book;
book.name = name;
book.author = author;
book.num = num;
books.push_back(book);
// 更新关键字索引
int index = books.size() - 1;
string keyword = name + author;
for (int i = 0; i < keyword.length(); i++) {
string sub = keyword.substr(i, 1);
if (index.find(sub) == index.end()) {
index[sub] = vector<int>();
}
index[sub].push_back(index);
}
}
// 根据名称和作者搜索图书
void searchBook(string name, string author) {
string keyword = name + author;
vector<int> result;
for (int i = 0; i < keyword.length(); i++) {
string sub = keyword.substr(i, 1);
if (index.find(sub) != index.end()) {
for (int j = 0; j < index[sub].size(); j++) {
int idx = index[sub][j];
if (books[idx].name == name && books[idx].author == author) {
result.push_back(idx);
}
}
}
}
if (result.empty()) {
cout << "没有找到相关图书" << endl;
} else {
cout << "找到了" << result.size() << "本相关图书" << endl;
for (int i = 0; i < result.size(); i++) {
cout << "名称:" << books[result[i]].name << endl;
cout << "作者:" << books[result[i]].author << endl;
cout << "数量:" << books[result[i]].num << endl;
cout << endl;
}
}
}
// 根据名称排序图书
bool cmpByName(Book a, Book b) {
return a.name < b.name;
}
// 根据作者排序图书
bool cmpByAuthor(Book a, Book b) {
return a.author < b.author;
}
// 显示所有图书
void showBooks() {
cout << "共有" << books.size() << "本图书" << endl;
for (int i = 0; i < books.size(); i++) {
cout << "名称:" << books[i].name << endl;
cout << "作者:" << books[i].author << endl;
cout << "数量:" << books[i].num << endl;
cout << endl;
}
}
int main() {
// 添加几本图书
addBook("C++程序设计", "谭浩强", 10);
addBook("C++高级编程", "侯捷", 5);
addBook("Java核心技术", "Cay S. Horstmann, Gary Cornell", 3);
// 按名称排序
sort(books.begin(), books.end(), cmpByName);
// 显示所有图书
showBooks();
// 根据名称和作者搜索图书
searchBook("C++程序设计", "谭浩强");
return 0;
}
```
该示例代码可以实现添加图书、按名称排序、按作者排序、搜索图书等功能。其中用vector存储图书信息,用map存储关键字索引。
当添加图书时,会将图书信息存储到vector中,并且会更新关键字索引。当搜索图书时,会根据名称和作者的关键字搜索图书,并且会输出搜索结果。当按名称排序或按作者排序时,使用algorithm库中的sort函数来实现排序功能。当显示所有图书时,会遍历vector中的所有图书信息,并且会输出每本图书的名称、作者和数量。
阅读全文
相关推荐
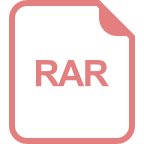
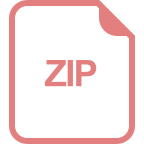
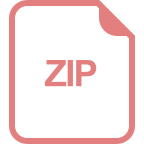
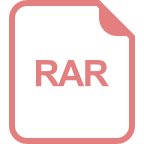
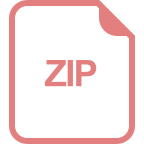
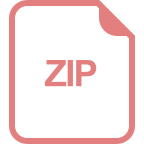
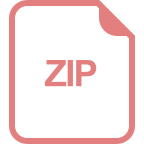
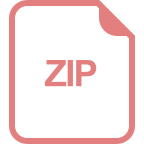

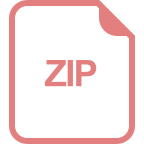
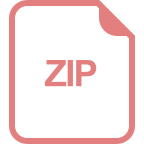
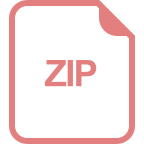
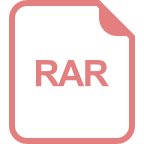
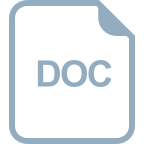
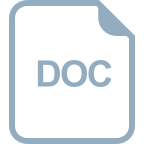
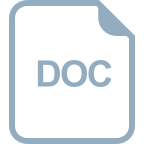
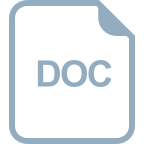
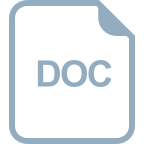