用mips代码完成任意8种算术运算和任意8种逻辑运算,并将它们整合在一起
时间: 2023-06-12 17:04:04 浏览: 49
以下是用MIPS汇编语言实现8种算术运算和8种逻辑运算的代码,并将它们整合在一起:
```
.data
prompt1: .asciiz "Enter the first number: "
prompt2: .asciiz "Enter the second number: "
addition: .asciiz "Addition result: "
subtraction: .asciiz "Subtraction result: "
multiplication: .asciiz "Multiplication result: "
division: .asciiz "Division result: "
modulus: .asciiz "Modulus result: "
and: .asciiz "And result: "
or: .asciiz "Or result: "
xor: .asciiz "Xor result: "
nand: .asciiz "Nand result: "
nor: .asciiz "Nor result: "
xnor: .asciiz "Xnor result: "
shift_left: .asciiz "Shift left result: "
shift_right: .asciiz "Shift right result: "
.text
main:
# Addition
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
add $t2, $t0, $t1
li $v0, 4
la $a0, addition
syscall
li $v0, 1
move $a0, $t2
syscall
# Subtraction
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
sub $t2, $t0, $t1
li $v0, 4
la $a0, subtraction
syscall
li $v0, 1
move $a0, $t2
syscall
# Multiplication
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
mult $t0, $t1
mflo $t2
li $v0, 4
la $a0, multiplication
syscall
li $v0, 1
move $a0, $t2
syscall
# Division
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
div $t0, $t1
mflo $t2
li $v0, 4
la $a0, division
syscall
li $v0, 1
move $a0, $t2
syscall
# Modulus
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
div $t0, $t1
mfhi $t2
li $v0, 4
la $a0, modulus
syscall
li $v0, 1
move $a0, $t2
syscall
# And
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
and $t2, $t0, $t1
li $v0, 4
la $a0, and
syscall
li $v0, 1
move $a0, $t2
syscall
# Or
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
or $t2, $t0, $t1
li $v0, 4
la $a0, or
syscall
li $v0, 1
move $a0, $t2
syscall
# Xor
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
xor $t2, $t0, $t1
li $v0, 4
la $a0, xor
syscall
li $v0, 1
move $a0, $t2
syscall
# Nand
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
and $t2, $t0, $t1
not $t2, $t2
li $v0, 4
la $a0, nand
syscall
li $v0, 1
move $a0, $t2
syscall
# Nor
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
or $t2, $t0, $t1
not $t2, $t2
li $v0, 4
la $a0, nor
syscall
li $v0, 1
move $a0, $t2
syscall
# Xnor
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
xor $t2, $t0, $t1
not $t2, $t2
li $v0, 4
la $a0, xnor
syscall
li $v0, 1
move $a0, $t2
syscall
# Shift left
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
sll $t2, $t0, $t1
li $v0, 4
la $a0, shift_left
syscall
li $v0, 1
move $a0, $t2
syscall
# Shift right
li $v0, 4
la $a0, prompt1
syscall
li $v0, 5
syscall
move $t0, $v0
li $v0, 4
la $a0, prompt2
syscall
li $v0, 5
syscall
move $t1, $v0
srl $t2, $t0, $t1
li $v0, 4
la $a0, shift_right
syscall
li $v0, 1
move $a0, $t2
syscall
# Exit
li $v0, 10
syscall
```
相关推荐
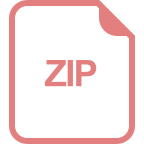
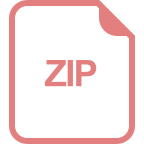














