2. 检查word文档的连续重复字,例如“用户的的资料”或“需要需要用户输入”之类的情况.(使用两种方法,其中一种为正则表达式)python代码
时间: 2024-03-17 19:46:17 浏览: 243
方法一:使用字符串操作
下面是使用字符串操作检查 Word 文档中连续重复字的 Python 代码示例:
```python
def find_duplicate_words(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
words = content.split()
duplicates = []
for i in range(1, len(words)):
if words[i] == words[i-1]:
if words[i] not in duplicates:
duplicates.append(words[i])
return duplicates
if __name__ == '__main__':
file_path = 'test.docx'
duplicates = find_duplicate_words(file_path)
if len(duplicates) == 0:
print('No duplicate words found.')
else:
print('Duplicate words found: ' + ', '.join(duplicates))
```
在这个示例中,我们定义了一个 find_duplicate_words 函数来检查 Word 文档中的连续重复字。函数接受一个参数,即要检查的 Word 文档的路径。函数首先读取文档内容,并使用 split 方法将文档内容按照单词划分成一个列表。然后,函数遍历列表,检查相邻两个单词是否相同。如果相同,则将这个单词添加到重复单词列表中。最后,函数返回重复单词列表。
在主函数中,我们可以调用 find_duplicate_words 函数来检查 Word 文档中的连续重复字。如果没有重复字,则输出“No duplicate words found.”;否则,输出所有重复字。
方法二:使用正则表达式
下面是使用正则表达式检查 Word 文档中连续重复字的 Python 代码示例:
```python
import re
def find_duplicate_words(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
pattern = r'\b(\w+)\s+\1\b'
duplicates = re.findall(pattern, content)
return duplicates
if __name__ == '__main__':
file_path = 'test.docx'
duplicates = find_duplicate_words(file_path)
if len(duplicates) == 0:
print('No duplicate words found.')
else:
print('Duplicate words found: ' + ', '.join(duplicates))
```
在这个示例中,我们使用了 Python 自带的 re 模块来检查 Word 文档中的连续重复字。首先,我们定义了一个 find_duplicate_words 函数来检查 Word 文档中的连续重复字。函数接受一个参数,即要检查的 Word 文档的路径。函数首先读取文档内容,并使用正则表达式查找连续重复字。具体地,我们使用了一个正则表达式 \b(\w+)\s+\1\b,其中 \b 表示单词边界,(\w+) 表示一个或多个单词字符,\s+ 表示一个或多个空格字符,\1 表示与第一个捕获组匹配的字符串。最后,我们使用 re.findall 方法查找所有匹配的字符串,并将它们添加到重复单词列表中。
在主函数中,我们可以调用 find_duplicate_words 函数来检查 Word 文档中的连续重复字。如果没有重复字,则输出“No duplicate words found.”;否则,输出所有重复字。
阅读全文
相关推荐
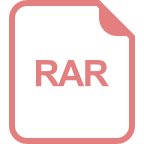
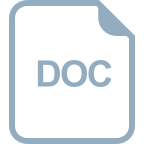
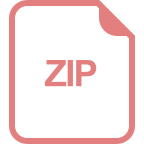
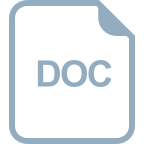
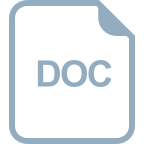
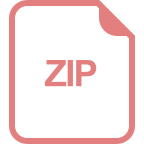
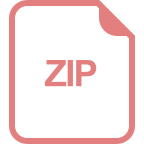
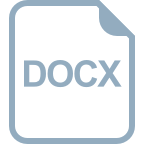
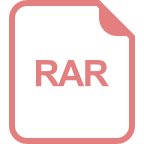
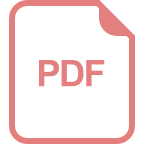
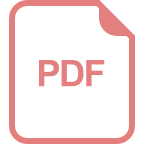
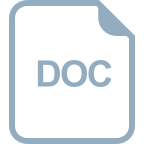
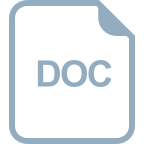
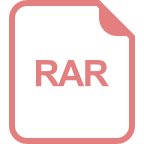
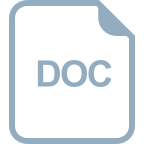