写一个基于J2ME的贪吃蛇游戏,可以在手机上或者模拟器上运行,不基于javax,简单java代码
时间: 2024-03-06 08:46:51 浏览: 119
好的,下面是一个基于J2ME的简单贪吃蛇游戏,使用了Canvas类来绘制游戏界面和处理用户输入。这个游戏可以在支持J2ME平台的手机上或模拟器上运行。
```
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Font;
import javax.microedition.lcdui.Graphics;
import javax.microedition.lcdui.TextField;
import javax.microedition.midlet.MIDlet;
import javax.microedition.midlet.MIDletStateChangeException;
public class SnakeGame extends MIDlet implements CommandListener {
private Display display;
private SnakeCanvas canvas;
private Command startCommand, exitCommand;
private boolean running;
public SnakeGame() {
display = Display.getDisplay(this);
canvas = new SnakeCanvas();
startCommand = new Command("Start", Command.OK, 1);
exitCommand = new Command("Exit", Command.EXIT, 1);
canvas.addCommand(startCommand);
canvas.addCommand(exitCommand);
canvas.setCommandListener(this);
running = false;
}
protected void startApp() throws MIDletStateChangeException {
display.setCurrent(canvas);
}
protected void pauseApp() {}
protected void destroyApp(boolean unconditional) throws MIDletStateChangeException {}
public void commandAction(Command c, Displayable d) {
if (c == startCommand) {
canvas.startGame();
} else if (c == exitCommand) {
try {
destroyApp(false);
notifyDestroyed();
} catch (MIDletStateChangeException e) {
e.printStackTrace();
}
}
}
private class SnakeCanvas extends Canvas implements Runnable {
private static final int WIDTH = getWidth();
private static final int HEIGHT = getHeight();
private static final int BLOCK_SIZE = 10;
private static final int GAME_WIDTH = WIDTH / BLOCK_SIZE;
private static final int GAME_HEIGHT = HEIGHT / BLOCK_SIZE;
private static final int MAX_SCORE = GAME_WIDTH * GAME_HEIGHT - 1;
private int[] snakeX, snakeY;
private int score;
private int foodX, foodY;
private int direction;
private boolean gameOver;
private boolean running;
private Thread gameThread;
public SnakeCanvas() {
setFullScreenMode(true);
snakeX = new int[MAX_SCORE];
snakeY = new int[MAX_SCORE];
score = 0;
direction = 0;
gameOver = false;
running = false;
gameThread = null;
}
public void startGame() {
if (!running) {
running = true;
gameOver = false;
score = 0;
direction = 0;
generateFood();
for (int i = 0; i < 3; i++) {
snakeX[i] = GAME_WIDTH / 2 + i;
snakeY[i] = GAME_HEIGHT / 2;
}
gameThread = new Thread(this);
gameThread.start();
}
}
public void run() {
while (running && score < MAX_SCORE && !gameOver) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
moveSnake();
checkCollision();
repaint();
}
gameOver = true;
running = false;
gameThread = null;
}
public void paint(Graphics g) {
g.setColor(0xFFFFFF);
g.fillRect(0, 0, WIDTH, HEIGHT);
g.setColor(0x000000);
g.drawRect(0, 0, WIDTH, HEIGHT);
g.drawString("Score: " + score, 0, 0, Graphics.TOP | Graphics.LEFT);
if (gameOver) {
g.drawString("Game Over", WIDTH / 2, HEIGHT / 2, Graphics.HCENTER | Graphics.BASELINE);
} else {
g.fillRect(foodX * BLOCK_SIZE, foodY * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
for (int i = 0; i < score; i++) {
g.fillRect(snakeX[i] * BLOCK_SIZE, snakeY[i] * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
protected void keyPressed(int keyCode) {
switch (keyCode) {
case KEY_LEFT:
if (direction != 1) {
direction = 3;
}
break;
case KEY_RIGHT:
if (direction != 3) {
direction = 1;
}
break;
case KEY_UP:
if (direction != 2) {
direction = 0;
}
break;
case KEY_DOWN:
if (direction != 0) {
direction = 2;
}
break;
}
}
private void generateFood() {
boolean valid = false;
while (!valid) {
foodX = (int) Math.floor(Math.random() * GAME_WIDTH);
foodY = (int) Math.floor(Math.random() * GAME_HEIGHT);
valid = true;
for (int i = 0; i < score; i++) {
if (snakeX[i] == foodX && snakeY[i] == foodY) {
valid = false;
break;
}
}
}
}
private void moveSnake() {
int dx = 0, dy = 0;
switch (direction) {
case 0:
dy = -1;
break;
case 1:
dx = 1;
break;
case 2:
dy = 1;
break;
case 3:
dx = -1;
break;
}
int newX = snakeX[0] + dx;
int newY = snakeY[0] + dy;
for (int i = score; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
snakeX[0] = newX;
snakeY[0] = newY;
if (newX == foodX && newY == foodY) {
score++;
generateFood();
}
}
private void checkCollision() {
if (snakeX[0] < 0 || snakeX[0] >= GAME_WIDTH || snakeY[0] < 0 || snakeY[0] >= GAME_HEIGHT) {
gameOver = true;
}
for (int i = 1; i < score; i++) {
if (snakeX[i] == snakeX[0] && snakeY[i] == snakeY[0]) {
gameOver = true;
break;
}
}
}
}
}
```
这个游戏使用了Canvas类来绘制游戏界面和处理用户输入,其中SnakeCanvas类是一个继承自Canvas的内部类,它实现了游戏逻辑和绘制游戏界面的方法。在SnakeCanvas的构造函数中,我们初始化了一些游戏变量,如蛇的长度、方向和位置,以及食物的位置。在startGame()方法中,我们通过启动一个新的线程来运行游戏逻辑。在run()方法中,我们处理蛇的移动、碰撞检测和界面重绘。
在SnakeCanvas类中,我们还重写了Canvas的keyPressed()方法来处理用户输入,根据用户输入的方向键来改变蛇的方向。在paint()方法中,我们绘制了游戏界面,包括蛇、食物和分数。
阅读全文
相关推荐
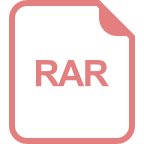
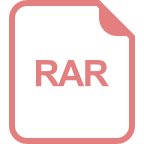
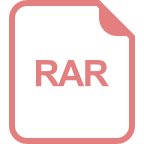
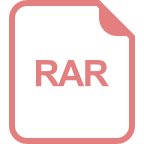
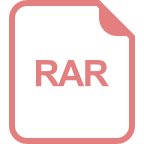
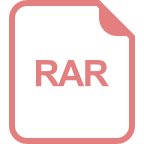
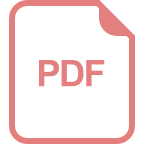
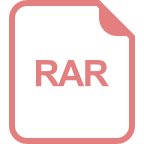
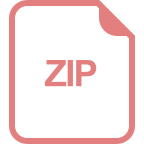
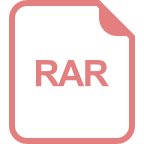
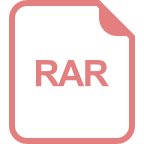
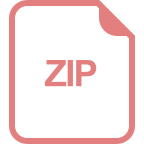
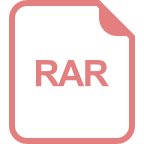
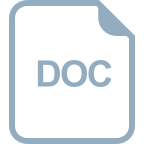
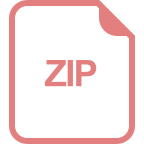
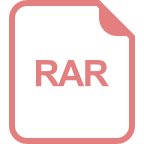
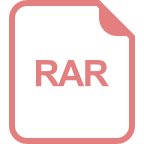