c语言编辑框代码
时间: 2023-08-12 07:22:31 浏览: 76
下面是一个简单的C语言编辑框的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
char ch;
char filename[100];
printf("请输入文件名: ");
scanf("%s", filename);
// 打开文件
fp = fopen(filename, "r");
// 读取并输出文件内容
if (fp) {
while ((ch = fgetc(fp)) != EOF) {
printf("%c", ch);
}
fclose(fp);
} else {
printf("无法打开文件 %s!\n", filename);
exit(1);
}
return 0;
}
```
这个程序会要求用户输入一个文件名,然后打开该文件并输出其中的内容。你可以根据自己的需要进行修改和扩展。
相关问题
c语言编辑框
为了在C语言中创建编辑框,你可以使用Windows API中的CreateWindow函数和Edit类。以下是一个简单示例:
```c
#include <windows.h>
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
// Register the window class.
const char CLASS_NAME[] = "MyClass";
WNDCLASS wc = { };
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
// Create the window.
HWND hwnd = CreateWindowEx(
0, // Optional window styles.
CLASS_NAME, // Window class
"C语言编辑框", // Window text
WS_OVERLAPPEDWINDOW, // Window style
// Size and position
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, // Parent window
NULL, // Menu
hInstance, // Instance handle
NULL // Additional application data
);
if (hwnd == NULL)
{
return 0;
}
ShowWindow(hwnd, nCmdShow);
// Run the message loop.
MSG msg = { };
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
// Handle other messages.
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
```
这将创建一个空白窗口。要在该窗口中添加编辑框,请在WndProc函数中添加以下代码:
```c
case WM_CREATE:
{
HWND hwndEdit = CreateWindowEx(
WS_EX_CLIENTEDGE, // Optional styles
"EDIT", // Edit class
"", // Text
WS_CHILD | WS_VISIBLE | ES_MULTILINE | ES_AUTOVSCROLL | ES_AUTOHSCROLL,
0, 0, 100, 100, // Position and size
hwnd, // Parent window
NULL, // No menu
GetModuleHandle(NULL), // Instance handle
NULL // Pointer to extra data
);
if (hwndEdit == NULL)
{
MessageBox(hwnd, "Could not create edit box.", "Error", MB_OK | MB_ICONERROR);
}
}
break;
```
这将在窗口中创建一个具有多行文本编辑功能的编辑框。你可以在CreateWindowEx函数中通过修改样式和位置来调整编辑框的大小和外观。
C语言编辑器贪吃蛇代码
在C语言中编写贪吃蛇游戏需要涉及图形界面的绘制以及基本的游戏逻辑控制。由于C语言不是最直观的图形化编程语言,通常我们会选择使用一些支持窗口和绘图功能的库,比如ncurses或者SFML等。以下是一个非常简化的贪吃蛇代码框架:
```c
#include <ncurses.h> // 使用ncurses库
#define MAX_WIDTH 80
#define MAX_HEIGHT 40
#define SNAKE_SIZE 10
#define FOOD_SIZE 1
void draw_snake(int x[], int y[], int length);
void eat_food(int snake[], int &length, int screen);
void move_snake(int snake[], int &direction);
bool check_collision(int snake[], int width, int height);
int main() {
initscr(); // 初始化ncurses环境
curs_set(0); // 隐藏光标
// 初始化蛇的位置、方向和其他变量...
while(1) { // 游戏主循环
chomp(); // 检查用户输入并处理
move_snake(snake, direction);
eat_food(snake, length, screen);
draw_snake(snake, ...); // 绘制蛇和食物
refresh(); // 更新屏幕
if(check_collision(snake, ..., ...)) break; // 如果发生碰撞,退出游戏
}
endwin(); // 关闭ncurses环境
return 0;
}
// 其他辅助函数如draw_snake、eat_food等
```
这只是一个起始点,实际编写会涉及到更多的细节,如玩家移动、食物生成、碰撞检测等。每个函数都需要详细设计和实现。
阅读全文
相关推荐

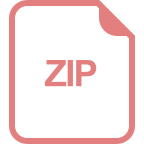





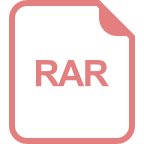

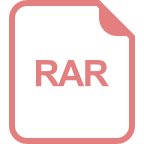



