C#web显示SQL表代码
时间: 2024-01-09 11:04:37 浏览: 37
以下是一个简单的 C# Web 应用程序,用于显示 SQL 表中的数据。
1. 在 Visual Studio 中创建一个新的 ASP.NET Web 应用程序项目。
2. 在项目中添加一个新的页面,例如 Default.aspx。
3. 在 Default.aspx 中添加一个 GridView 控件,用于显示 SQL 表中的数据。
```html
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs"
Inherits="WebApplication1._Default" %>
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server"></asp:GridView>
</div>
</form>
</body>
</html>
```
4. 在 Default.aspx.cs 中编写代码,用于连接到 SQL 数据库并将数据绑定到 GridView 控件上。
```csharp
using System;
using System.Data.SqlClient;
namespace WebApplication1
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
string connectionString = "Data Source=(local);Initial Catalog=YourDatabaseName;Integrated Security=True";
string query = "SELECT * FROM YourTableName";
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(query, connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
GridView1.DataSource = reader;
GridView1.DataBind();
reader.Close();
}
}
}
}
```
请注意,您需要使用正确的数据库名称和表名称来替换代码中的 "YourDatabaseName" 和 "YourTableName"。另外,您可能需要更改 connectionString 变量以反映您的服务器名称和身份验证方法。
相关推荐
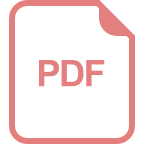
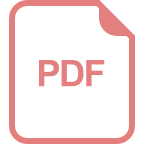
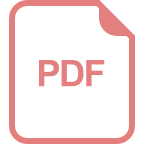














