用c语言使用递归方法实现,向终端输出指定目录下所有子目录的文件名
时间: 2024-03-10 14:50:58 浏览: 147
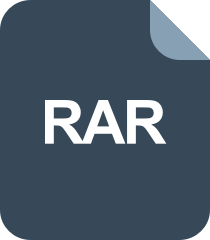
C# 用递归的方式查找指定文件夹下的所有子目录

好的,下面是使用递归方法实现输出指定目录下所有子目录的文件名的 C 语言代码示例:
```c
#include <stdio.h>
#include <dirent.h>
#include <sys/stat.h>
#include <string.h>
void print_files(char *dir_path)
{
DIR *dir;
struct dirent *entry;
struct stat statbuf;
char file_path[256];
if ((dir = opendir(dir_path)) == NULL) {
fprintf(stderr, "Error: cannot open directory %s\n", dir_path);
return;
}
while ((entry = readdir(dir)) != NULL) {
sprintf(file_path, "%s/%s", dir_path, entry->d_name);
if (lstat(file_path, &statbuf) < 0) {
fprintf(stderr, "Error: cannot stat file %s\n", file_path);
continue;
}
if (S_ISDIR(statbuf.st_mode)) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
printf("directory: %s\n", file_path);
print_files(file_path);
} else {
printf("file: %s\n", file_path);
}
}
closedir(dir);
}
int main(int argc, char *argv[])
{
if (argc < 2) {
fprintf(stderr, "Usage: %s <directory>\n", argv[0]);
return 1;
}
print_files(argv[1]);
return 0;
}
```
在这个示例中,我们使用 `opendir()` 函数打开指定目录,然后使用 `readdir()` 函数遍历目录中的所有文件和子目录。对于每个文件或子目录,我们使用 `lstat()` 函数获取其详细属性信息,判断其是否为子目录。如果是子目录,则递归调用 `print_files()` 函数,继续遍历该子目录下的所有文件和子目录;如果是普通文件,则直接输出文件路径。
需要注意的是,在递归调用 `print_files()` 函数时,需要传入子目录的路径,而不是子目录的文件名。另外,为了防止无限递归,我们需要排除子目录中的 `.` 和 `..` 文件。
最后,在 `main()` 函数中,我们检查是否传入了指定目录的路径参数,如果没有则输出使用方法。然后调用 `print_files()` 函数,传入指定目录的路径即可。
阅读全文
相关推荐

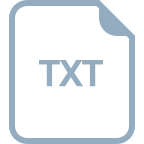
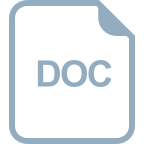
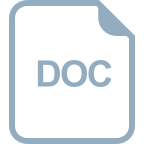

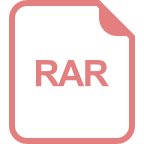
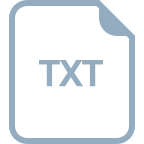
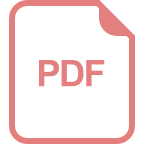
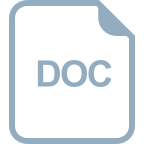
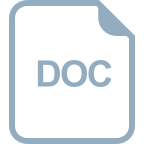
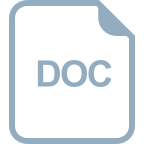
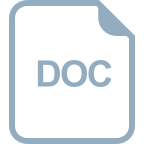


