SSM框架分页展示Hdfs文件列表上传文件,删除文件,下载文件前后端代码
时间: 2023-06-12 18:05:15 浏览: 50
1. 分页展示Hdfs文件列表
前端代码:
```html
<!-- 分页插件 -->
<link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script>
<script src="https://cdn.bootcss.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<table class="table table-hover">
<thead>
<tr>
<th>#</th>
<th>文件名</th>
<th>大小</th>
<th>修改时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${hdfsFiles}" var="hdfsFile" varStatus="status">
<tr>
<td>${status.index+1}</td>
<td><a href="#">${hdfsFile.name}</a></td>
<td>${hdfsFile.size}</td>
<td>${hdfsFile.modificationTime}</td>
<td>
<button class="btn btn-danger" onclick="deleteFile('${hdfsFile.path}')">删除</button>
<button class="btn btn-success" onclick="downloadFile('${hdfsFile.path}')">下载</button>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<div class="text-center">
<ul class="pagination">
<c:choose>
<c:when test="${pageInfo.pageNum == 1}">
<li class="disabled"><a href="#">首页</a></li>
<li class="disabled"><a href="#">上一页</a></li>
</c:when>
<c:otherwise>
<li><a href="?pageNum=1">首页</a></li>
<li><a href="?pageNum=${pageInfo.prePage}">上一页</a></li>
</c:otherwise>
</c:choose>
<c:forEach begin="${pageInfo.navigateFirstPage}" end="${pageInfo.navigateLastPage}" var="i">
<c:choose>
<c:when test="${i == pageInfo.pageNum}">
<li class="active"><a href="#">${i}</a></li>
</c:when>
<c:otherwise>
<li><a href="?pageNum=${i}">${i}</a></li>
</c:otherwise>
</c:choose>
</c:forEach>
<c:choose>
<c:when test="${pageInfo.pageNum == pageInfo.pages}">
<li class="disabled"><a href="#">下一页</a></li>
<li class="disabled"><a href="#">尾页</a></li>
</c:when>
<c:otherwise>
<li><a href="?pageNum=${pageInfo.nextPage}">下一页</a></li>
<li><a href="?pageNum=${pageInfo.pages}">尾页</a></li>
</c:otherwise>
</c:choose>
</ul>
</div>
```
后端代码:
```java
@RequestMapping("/listFiles")
public ModelAndView listFiles(@RequestParam(defaultValue = "1") Integer pageNum, @RequestParam(defaultValue = "10") Integer pageSize) throws Exception {
ModelAndView mv = new ModelAndView("fileList");
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), new Configuration(), "root");
RemoteIterator<LocatedFileStatus> fileStatusListIterator = fs.listFiles(new Path("/"), true);
List<HdfsFile> hdfsFiles = new ArrayList<>();
while (fileStatusListIterator.hasNext()) {
LocatedFileStatus fileStatus = fileStatusListIterator.next();
HdfsFile hdfsFile = new HdfsFile();
hdfsFile.setName(fileStatus.getPath().getName());
hdfsFile.setPath(fileStatus.getPath().toString());
hdfsFile.setSize(fileStatus.getLen());
hdfsFile.setModificationTime(fileStatus.getModificationTime());
hdfsFiles.add(hdfsFile);
}
PageHelper.startPage(pageNum, pageSize);
PageInfo<HdfsFile> pageInfo = new PageInfo<>(hdfsFiles);
mv.addObject("hdfsFiles", pageInfo.getList());
mv.addObject("pageInfo", pageInfo);
return mv;
}
```
2. 上传文件
前端代码:
```html
<form action="${pageContext.request.contextPath}/uploadFile" method="post" enctype="multipart/form-data">
<div class="form-group">
<label>选择文件</label>
<input type="file" name="file">
</div>
<button type="submit" class="btn btn-primary">上传</button>
</form>
```
后端代码:
```java
@RequestMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file) throws IOException, URISyntaxException {
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), new Configuration(), "root");
Path dst = new Path("/" + file.getOriginalFilename());
FSDataOutputStream outputStream = fs.create(dst);
IOUtils.copyBytes(file.getInputStream(), outputStream, 4096, true);
return "redirect:/listFiles";
}
```
3. 删除文件
前端代码:
```html
<button class="btn btn-danger" onclick="deleteFile('${hdfsFile.path}')">删除</button>
```
后端代码:
```java
@RequestMapping("/deleteFile")
public String deleteFile(String path) throws IOException, URISyntaxException {
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), new Configuration(), "root");
Path dst = new Path(path);
fs.delete(dst, true);
return "redirect:/listFiles";
}
```
4. 下载文件
前端代码:
```html
<button class="btn btn-success" onclick="downloadFile('${hdfsFile.path}')">下载</button>
```
后端代码:
```java
@RequestMapping("/downloadFile")
public ResponseEntity<byte[]> downloadFile(String path) throws IOException, URISyntaxException {
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), new Configuration(), "root");
Path dst = new Path(path);
FSDataInputStream inputStream = fs.open(dst);
byte[] bytes = IOUtils.toByteArray(inputStream);
HttpHeaders headers = new HttpHeaders();
headers.setContentDispositionFormData("attachment", dst.getName());
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
return new ResponseEntity<>(bytes, headers, HttpStatus.CREATED);
}
```
相关推荐
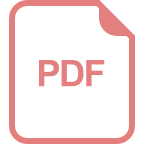
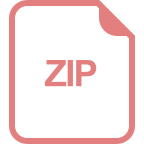
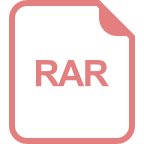
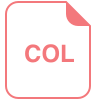
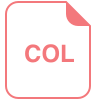
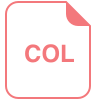
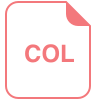
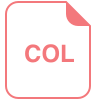


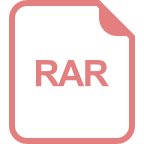
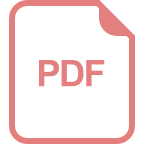
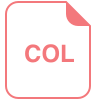
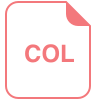
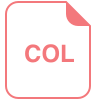
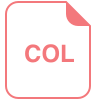
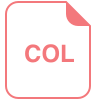