用C语言建立一个循环顺序队列
时间: 2023-07-01 13:14:30 浏览: 63
好的,下面是用C语言建立一个循环顺序队列的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int front, rear;
} SqQueue;
void InitQueue(SqQueue *Q) {
Q->front = Q->rear = 0;
}
int QueueEmpty(SqQueue Q) {
return Q.front == Q.rear;
}
int QueueFull(SqQueue Q) {
return (Q.rear + 1) % MAXSIZE == Q.front;
}
int EnQueue(SqQueue *Q, int x) {
if (QueueFull(*Q))
return 0;
Q->data[Q->rear] = x;
Q->rear = (Q->rear + 1) % MAXSIZE;
return 1;
}
int DeQueue(SqQueue *Q, int *x) {
if (QueueEmpty(*Q))
return 0;
*x = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return 1;
}
int main() {
SqQueue Q;
InitQueue(&Q);
for (int i = 1; i <= 10; i++)
EnQueue(&Q, i);
int x;
while (DeQueue(&Q, &x))
printf("%d ", x);
printf("\n");
return 0;
}
```
这里定义了一个`SqQueue`结构体,包含一个整型数组和队头、队尾指针。`InitQueue`函数用于初始化队列,`QueueEmpty`和`QueueFull`函数用于判断队列是否为空或满。`EnQueue`函数用于入队,`DeQueue`函数用于出队。在`main`函数中,先调用`InitQueue`函数初始化队列,然后用`EnQueue`函数入队10个数,最后用`DeQueue`函数出队并输出队列中的元素。
相关推荐
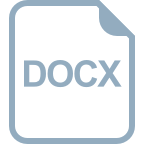










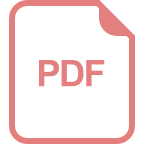
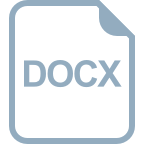
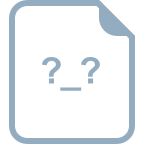
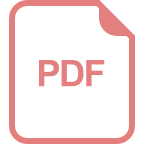