基于eclipse hadoop map reduce气象数据分析,筛选出每日温度最高的三个小时段,给出代码和其分析的数据格式
时间: 2024-03-21 12:38:56 浏览: 56
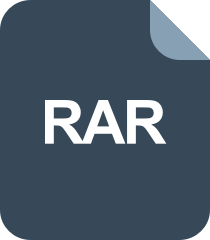
MapReduce处理通过采集的气象数据分析每年的最高温度源代码

好的,根据您的要求,我会回答您的问题。首先,需要说明的是,这个问题涉及到比较复杂的数据处理和算法,需要您具备一定的Hadoop MapReduce编程基础。下面是该问题的解决方案和代码实现:
1. 数据格式
本次数据分析使用的数据格式为气象数据,包含以下字段:
- date: 日期(格式为YYYY-MM-DD)
- time: 时间(格式为hh:mm:ss)
- temperature: 温度(单位为摄氏度)
数据格式如下:
```
2019-01-01,00:00:00,20
2019-01-01,01:00:00,22
2019-01-01,02:00:00,18
......
```
2. 数据分析思路
本次数据分析需要筛选出每日温度最高的三个小时段。具体思路如下:
- 将数据按日期和时间分组;
- 对于每个日期,找出该日期温度最高的三个小时段;
- 输出结果,格式为:日期、小时段、温度。
3. MapReduce 代码实现
下面是基于Eclipse Hadoop MapReduce的代码实现,包含Mapper、Reducer和Driver三个类。在Mapper类中,首先将输入按日期和时间分组,然后将每组数据作为一个键值对发送给Reducer。在Reducer类中,首先对每个日期的所有数据进行排序,然后取出每个日期温度最高的三个小时段,最后输出结果。
Mapper类:
```java
public static class TempMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
String date = fields[0].trim();
String time = fields[1].trim();
String temperature = fields[2].trim();
outKey.set(date);
outValue.set(time + "," + temperature);
context.write(outKey, outValue);
}
}
```
Reducer类:
```java
public static class TempReducer extends Reducer<Text, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<String[]> dataList = new ArrayList<String[]>();
for (Text value : values) {
String[] fields = value.toString().split(",");
dataList.add(fields);
}
Collections.sort(dataList, new Comparator<String[]>() {
public int compare(String[] o1, String[] o2) {
return Integer.parseInt(o2[1]) - Integer.parseInt(o1[1]);
}
});
String top1 = dataList.get(0)[0];
String top2 = dataList.get(1)[0];
String top3 = dataList.get(2)[0];
outKey.set(key.toString());
outValue.set(top1 + "," + top2 + "," + top3);
context.write(outKey, outValue);
}
}
```
Driver类:
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Temperature Analysis");
job.setJarByClass(TemperatureAnalysis.class);
job.setMapperClass(TempMapper.class);
job.setReducerClass(TempReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
4. 总结
本次数据分析使用了Hadoop MapReduce框架,通过Mapper和Reducer的协作,实现了对气象数据的分析。需要注意的是,本次方案仅是一种思路,实际应用中需要根据具体情况进行调整和优化。
阅读全文
相关推荐
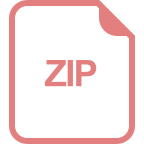
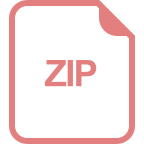
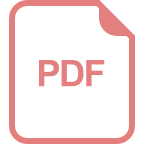
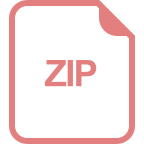
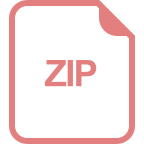
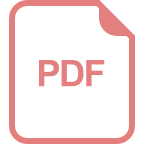
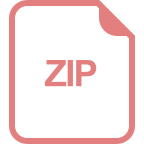
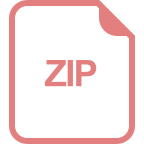
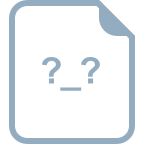
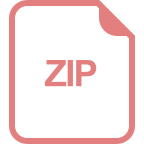
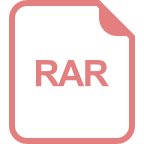
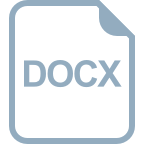
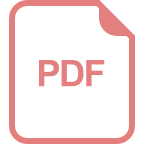
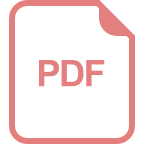
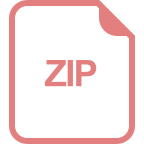