python将文件复制到指定文件夹里
时间: 2023-09-18 11:08:06 浏览: 45
你可以使用Python的shutil模块来复制文件到指定的文件夹里。以下是一个示例代码:
```python
import shutil
# 源文件路径
src_file = '/path/to/source/file.txt'
# 目标文件夹路径
dest_folder = '/path/to/destination/folder/'
# 复制文件
shutil.copy(src_file, dest_folder)
```
在这个示例中,`src_file` 是需要复制的文件路径,`dest_folder` 是目标文件夹路径。 `shutil.copy()` 函数将源文件复制到目标文件夹里。
相关问题
python将文件复制进指定文件夹
你可以使用shutil模块中的copy2()函数将文件复制到指定文件夹中。以下是一个示例代码:
```python
import shutil
import os
# 源文件路径
src_file = "file.txt"
# 目标文件夹路径
dst_folder = "C:/Users/User/Desktop/destination_folder"
# 确保目标文件夹存在
if not os.path.exists(dst_folder):
os.makedirs(dst_folder)
# 复制文件到目标文件夹中
shutil.copy2(src_file, dst_folder)
```
在这个示例中,我们首先指定源文件的路径(src_file)和目标文件夹的路径(dst_folder)。然后,我们使用os模块中的makedirs()函数创建目标文件夹(如果它不存在)。最后,我们使用shutil模块中的copy2()函数将源文件复制到目标文件夹中。
python将文件储存到指定文件夹
您可以使用Python的`shutil`模块将文件存储到指定文件夹。以下是一个示例代码:
```python
import shutil
# 源文件路径
source_file = 'path/to/source/file.txt'
# 目标文件夹路径
target_folder = 'path/to/target/folder/'
# 使用shutil模块的`move`函数将文件移动到目标文件夹
shutil.move(source_file, target_folder)
```
在上面的示例中,您需要将`source_file`变量设置为源文件的路径,将`target_folder`变量设置为目标文件夹的路径。然后,使用`shutil.move()`函数将文件从源路径移动到目标文件夹。
另外,如果您想将文件复制到目标文件夹而不是移动,可以使用`shutil.copy()`函数。示例如下:
```python
import shutil
# 源文件路径
source_file = 'path/to/source/file.txt'
# 目标文件夹路径
target_folder = 'path/to/target/folder/'
# 使用shutil模块的`copy`函数将文件复制到目标文件夹
shutil.copy(source_file, target_folder)
```
这样,源文件将被复制到目标文件夹中,而不会从原始位置移动。
阅读全文
相关推荐
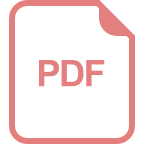












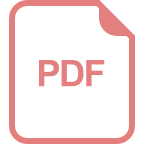
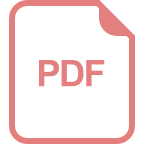