用C++设计一个航空订票系统‘
时间: 2024-03-26 10:38:20 浏览: 14
好的,以下是一个简单的航空订票系统的C++代码示例:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 定义航班类
class Flight {
public:
Flight(string name, string from, string to, double price, int capacity) :
name(name), from(from), to(to), price(price), capacity(capacity) {}
string getName() const { return name; }
string getFrom() const { return from; }
string getTo() const { return to; }
double getPrice() const { return price; }
int getCapacity() const { return capacity; }
private:
string name;
string from;
string to;
double price;
int capacity;
};
// 定义订票类
class Booking {
public:
Booking(string name, string flightName, int numTickets) :
name(name), flightName(flightName), numTickets(numTickets) {}
string getName() const { return name; }
string getFlightName() const { return flightName; }
int getNumTickets() const { return numTickets; }
private:
string name;
string flightName;
int numTickets;
};
// 定义系统类
class AirlineSystem {
public:
// 添加航班
void addFlight(Flight f) {
flights.push_back(f);
}
// 订票
bool bookFlight(Booking b) {
// 查找对应的航班
auto it = find_if(flights.begin(), flights.end(), [&](const Flight& f) {
return f.getName() == b.getFlightName();
});
if (it == flights.end()) {
return false;
}
// 检查是否有足够的座位
if (it->getCapacity() < b.getNumTickets()) {
return false;
}
// 更新座位数
it->capacity -= b.getNumTickets();
// 添加订单
bookings.push_back(b);
return true;
}
// 显示所有航班
void displayFlights() const {
for (const auto& f : flights) {
cout << "Flight " << f.getName() << " from " << f.getFrom() <<
" to " << f.getTo() << " price: " << f.getPrice() <<
" capacity: " << f.getCapacity() << endl;
}
}
// 显示所有订单
void displayBookings() const {
for (const auto& b : bookings) {
cout << "Booking for " << b.getName() << " on flight " <<
b.getFlightName() << " for " << b.getNumTickets() <<
" tickets" << endl;
}
}
private:
vector<Flight> flights;
vector<Booking> bookings;
};
int main() {
// 创建系统
AirlineSystem system;
// 添加航班
system.addFlight(Flight("AA123", "New York", "Los Angeles", 200, 100));
system.addFlight(Flight("UA456", "Chicago", "San Francisco", 300, 50));
system.addFlight(Flight("DL789", "Boston", "Miami", 150, 200));
// 显示所有航班
system.displayFlights();
// 订票
Booking b1("John", "AA123", 2);
Booking b2("Mary", "DL789", 1);
Booking b3("Tom", "UA456", 3);
bool success1 = system.bookFlight(b1);
bool success2 = system.bookFlight(b2);
bool success3 = system.bookFlight(b3);
if (success1 && success2 && success3) {
cout << "Bookings successful!" << endl;
}
else {
cout << "Bookings failed!" << endl;
}
// 显示所有订单
system.displayBookings();
return 0;
}
```
该系统使用三个类表示航班、订票和整个系统,利用向量存储所有航班和订单。可以使用 `addFlight` 添加航班,使用 `bookFlight` 订票,使用 `displayFlights` 和 `displayBookings` 显示所有航班和订单。
相关推荐
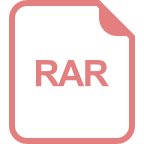









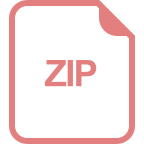
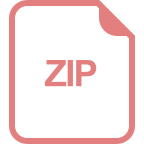
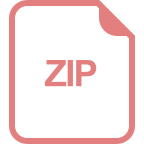
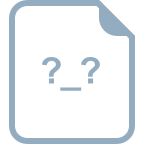