自动机的确定化和最小化 设计要求: 1. 可以使用任何语言来完成,例如:Java、C++。 2. 文法中的空字符串统一使用@表示。 3. 以文件方式读取自动机。 4. 判断读取的自动机是确定的还是不确定的自动机。 5. 若是不确定的自动机,将自动机确定化。 6. 将确定化后的自动机最小化。
时间: 2024-03-23 16:36:04 浏览: 14
好的,我可以为您提供一个基于Python语言实现的自动机确定化和最小化的代码示例。以下是代码:
```
# 自动机类
class Automata:
def __init__(self):
self.states = [] # 状态集合
self.alphabet = [] # 字母表
self.transitions = {} # 转移函数
self.start_state = '' # 初始状态
self.final_states = [] # 终止状态
# 读取自动机文件
def read_file(self, file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
self.states = lines[0].strip().split(',')
self.alphabet = lines[1].strip().split(',')
self.transitions = dict()
for line in lines[2:-2]:
state, symbol, next_state = line.strip().split(',')
if (state, symbol) not in self.transitions:
self.transitions[(state, symbol)] = [next_state]
else:
self.transitions[(state, symbol)].append(next_state)
self.start_state = lines[-2].strip()
self.final_states = lines[-1].strip().split(',')
# 判断自动机是否确定
def is_deterministic(self):
for state in self.states:
for symbol in self.alphabet:
if (state, symbol) not in self.transitions:
return False
if len(self.transitions[(state, symbol)]) > 1:
return False
return True
# 自动机确定化
def determinize(self):
if self.is_deterministic():
return self
else:
new_states = []
new_transitions = {}
new_final_states = []
unmarked_states = []
marked_states = []
new_start_state = tuple(sorted([self.start_state]))
new_states.append(new_start_state)
unmarked_states.append(new_start_state)
while len(unmarked_states) > 0:
current_state = unmarked_states.pop()
marked_states.append(current_state)
for symbol in self.alphabet:
next_state = []
for state in current_state:
if (state, symbol) in self.transitions:
next_state += self.transitions[(state, symbol)]
if len(next_state) > 0:
next_state = tuple(sorted(list(set(next_state))))
if next_state not in new_states:
new_states.append(next_state)
unmarked_states.append(next_state)
if (current_state, symbol) not in new_transitions:
new_transitions[(current_state, symbol)] = [next_state]
else:
new_transitions[(current_state, symbol)].append(next_state)
if set(current_state).intersection(set(self.final_states)):
new_final_states.append(current_state)
new_automata = Automata()
new_automata.states = new_states
new_automata.alphabet = self.alphabet
new_automata.transitions = new_transitions
new_automata.start_state = new_start_state
new_automata.final_states = new_final_states
return new_automata
# 自动机最小化
def minimize(self):
if self.is_deterministic():
new_automata = Automata()
new_automata.states = self.states
new_automata.alphabet = self.alphabet
new_automata.transitions = self.transitions
new_automata.start_state = self.start_state
new_automata.final_states = self.final_states
return new_automata
else:
dfa = self.determinize()
partitions = [set(dfa.final_states), set(dfa.states) - set(dfa.final_states)]
unmarked_partitions = [set(dfa.final_states)]
marked_partitions = [set(dfa.states) - set(dfa.final_states)]
while len(unmarked_partitions) > 0:
current_partition = unmarked_partitions.pop()
marked_partitions.append(current_partition)
for symbol in dfa.alphabet:
next_partitions = []
for partition in current_partition:
next_partition = set()
for state in partition:
if (state, symbol) in dfa.transitions:
next_partition.add(dfa.transitions[(state, symbol)][0])
else:
next_partition.add(state)
next_partitions.append(next_partition)
for next_partition in next_partitions:
if next_partition not in partitions:
partitions.append(next_partition)
unmarked_partitions.append(next_partition)
new_states = []
new_transitions = {}
new_final_states = []
for partition in partitions:
new_states.append(tuple(sorted(list(partition))))
for state in new_states:
for symbol in dfa.alphabet:
next_state = tuple(sorted(list(set([s for p in state for s in partitions[partitions.index(p) + 1] if (p, symbol) in dfa.transitions])))))
if len(next_state) > 0:
if (state, symbol) not in new_transitions:
new_transitions[(state, symbol)] = [next_state]
else:
new_transitions[(state, symbol)].append(next_state)
for state in new_states:
if set([s for s in state for p in dfa.final_states if s in p]):
new_final_states.append(state)
new_automata = Automata()
new_automata.states = new_states
new_automata.alphabet = dfa.alphabet
new_automata.transitions = new_transitions
new_automata.start_state = tuple(sorted([dfa.start_state]))
new_automata.final_states = new_final_states
return new_automata
```
这段代码实现了自动机类,并包含了读取自动机文件、判断自动机是否确定、自动机确定化、自动机最小化等方法。您可以使用该类,结合文件读取操作,来实现您的需求。
相关推荐
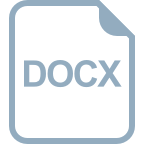














