python 读写json文件
时间: 2023-10-04 14:12:02 浏览: 106
你可以使用Python的内置模块`json`来读写JSON文件。下面是一个示例:
```python
import json
# 读取JSON文件
with open('data.json', 'r') as file:
data = json.load(file)
# 修改数据
data['name'] = 'Alice'
data['age'] = 25
# 写入JSON文件
with open('data.json', 'w') as file:
json.dump(data, file)
```
在这个示例中,我们首先使用`json.load()`函数从文件中加载JSON数据并存储在变量`data`中。然后,我们对`data`进行修改,例如修改"name"和"age"字段的值。最后,我们使用`json.dump()`函数将更新后的数据写回到文件中。
记得将`data.json`替换为你自己的JSON文件路径。
相关问题
Python读写json文件
在Python中,你可以使用内置的json模块来读写JSON文件。下面是一个简单的例子:
```python
import json
# 读取JSON文件
def read_json_file(file_path):
with open(file_path, 'r') as file:
data = json.load(file)
return data
# 写入JSON文件
def write_json_file(data, file_path):
with open(file_path, 'w') as file:
json.dump(data, file, indent=4)
# 示例:读取JSON文件
json_data = read_json_file('data.json')
print(json_data)
# 示例:写入JSON文件
data = {'name': 'John', 'age': 30, 'city': 'New York'}
write_json_file(data, 'output.json')
```
上述代码中,`read_json_file`函数用于从JSON文件中读取数据,`write_json_file`函数用于将数据写入JSON文件。你可以根据需要修改文件路径和文件名。
python 读写json
Python中读写JSON格式的数据可以使用内置的json模块,这里给出一些基本的示例代码。
1. 读取JSON文件
假设我们有一个名为data.json的JSON文件,其内容如下:
```json
{
"name": "John",
"age": 30,
"isMarried": true,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
我们可以使用以下代码读取该文件并将其解析为Python对象:
```python
import json
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
```
输出结果为:
```python
{
"name": "John",
"age": 30,
"isMarried": true,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
2. 将Python对象写入JSON文件
假设我们有一个名为data的Python对象,其内容如下:
```python
data = {
"name": "John",
"age": 30,
"isMarried": True,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
我们可以使用以下代码将该Python对象写入名为output.json的JSON文件:
```python
import json
with open('output.json', 'w') as f:
json.dump(data, f)
```
这将在当前工作目录下创建一个名为output.json的文件,并将Python对象data的JSON表示写入其中。
以上就是基本的JSON读写操作,希望能对你有所帮助。
阅读全文
相关推荐
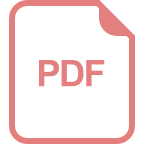
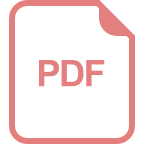
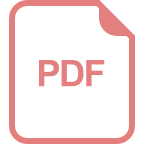
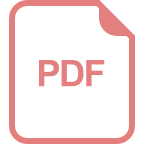






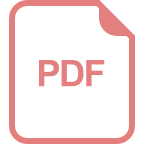
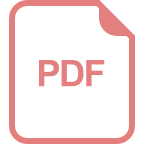