c#中文本框怎么在输入的是加密状态
时间: 2024-10-26 17:17:19 浏览: 18
在C#中,如果你想要在文本框(TextBox)中输入的内容默认是加密的,并显示为密文,你可以使用`System.Security.Cryptography`命名空间提供的加密算法。例如,可以使用`Aes`类进行对称加密。以下是基本步骤:
1. 首先,你需要创建一个加解密类,用于封装加密和解密操作:
```csharp
public static class EncryptionHelper
{
private static Aes aes = Aes.Create();
//...省略具体的初始化向量和密钥生成方法
public static string Encrypt(string plainText)
{
byte[] encryptedBytes = EncryptStringToBytes_Aes(plainText);
return Convert.ToBase64String(encryptedBytes);
}
public static string Decrypt(string cipherText)
{
byte[] cipherBytes = Convert.FromBase64String(cipherText);
return DecryptStringFromBytes_Aes(cipherBytes);
}
private static byte[] EncryptStringToBytes_Aes(string plainText)
{
//...在这里添加实际的加密操作
}
private static string DecryptStringFromBytes_Aes(byte[] cipherBytes)
{
//...在这里添加实际的解密操作
}
}
```
2. 在文本框的值改变事件中,对输入内容进行加密:
```csharp
private void textBox_TextChanged(object sender, EventArgs e)
{
string input = textBox.Text;
if (!string.IsNullOrEmpty(input))
{
textBox.Text = EncryptionHelper.Encrypt(input);
}
}
```
3. 当需要显示原始文本时,使用解密方法:
```csharp
private void button_Decrypt_Click(object sender, EventArgs e)
{
string encryptedInput = textBox.Text;
textBox.Text = EncryptionHelper.Decrypt(encryptedInput);
}
```
请注意,为了安全起见,你应该处理好密钥和初始向量的存储问题,以及在实际应用中使用更高级的加密模式和填充模式。
阅读全文
相关推荐
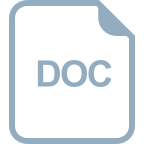
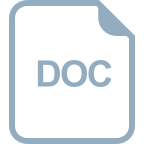
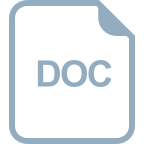
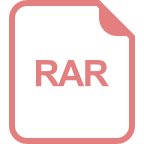
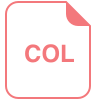
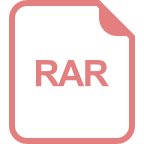
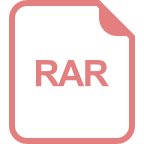
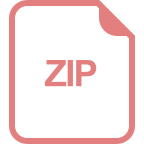
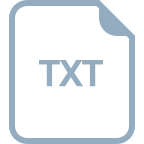
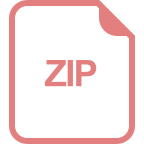
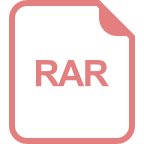
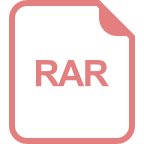
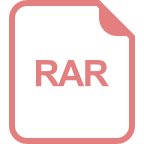
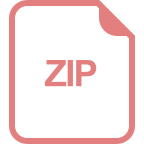
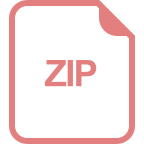
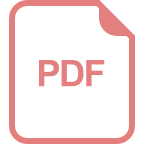
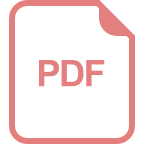
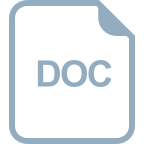
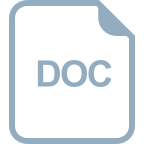