entries.stream().map
时间: 2024-11-16 15:12:31 浏览: 5
`entries.stream().map` 是Java Stream API中的一个操作,用于对集合(在这个情况下可能是Map的entry集)进行映射转换。Stream API提供了一种处理数据流的方式,使得我们可以对大量数据进行并行处理。
当你调用 `entries.stream()` 时,你会创建一个流(Stream),这个流包含了Map的所有条目(键值对)。`.map` 方法则接受一个函数作为参数,这个函数会对每个entry应用,通常用来转换entry的内容或结构。
例如,如果你有一个Map<String, Integer>,你可以使用 `.map` 来把所有的数值转换为它们的平方,或者把键转换为大写字符串。这里有个简单的例子:
```java
Map<String, Integer> map = new HashMap<>();
map.put("one", 1);
map.put("two", 2);
// 使用map方法,将每个Integer值转换为它的平方
List<Integer> squares = map.entrySet().stream()
.map(entry -> entry.getValue() * entry.getValue())
.collect(Collectors.toList());
```
这将会返回一个新的列表 `[1, 4]`,其中包含原始Map中每个键对应的值的平方。
相关问题
优化下面这段代码Map<String, List<Department>> listMap = all.stream().collect(Collectors.groupingBy(Department::getBigcode)); Set<Map.Entry<String, List<Department>>> entries = listMap.entrySet(); List<DepartmentVo> departmentVoList = new ArrayList<>(); for (Map.Entry<String, List<Department>> entry : entries) { DepartmentVo departmentVo = new DepartmentVo(); //大科室编号 String bigCode = entry.getKey(); List<Department> departmentList = entry.getValue(); //大科室名称 String bigName = departmentList.get(0).getBigname(); departmentVo.setDepcode(bigCode); departmentVo.setDepname(bigName); //接下来进行子列表操作 List<DepartmentVo> childDepartmentVoList = new ArrayList<>(); for (Department childDepartment : departmentList) { DepartmentVo childDepartmentVo = new DepartmentVo(); childDepartmentVo.setDepcode(childDepartment.getDepcode()); childDepartmentVo.setDepname(childDepartment.getDepname()); childDepartmentVoList.add(childDepartmentVo); } departmentVo.setChildren(childDepartmentVoList); departmentVoList.add(departmentVo); } return departmentVoList;
可以使用Stream API的flatMap操作来简化代码,如下所示:
```
List<DepartmentVo> departmentVoList = all.stream()
.collect(Collectors.groupingBy(Department::getBigcode))
.entrySet().stream()
.map(entry -> {
DepartmentVo departmentVo = new DepartmentVo();
String bigCode = entry.getKey();
List<Department> departmentList = entry.getValue();
String bigName = departmentList.get(0).getBigname();
departmentVo.setDepcode(bigCode);
departmentVo.setDepname(bigName);
List<DepartmentVo> childDepartmentVoList = departmentList.stream()
.map(childDepartment -> {
DepartmentVo childDepartmentVo = new DepartmentVo();
childDepartmentVo.setDepcode(childDepartment.getDepcode());
childDepartmentVo.setDepname(childDepartment.getDepname());
return childDepartmentVo;
})
.collect(Collectors.toList());
departmentVo.setChildren(childDepartmentVoList);
return departmentVo;
})
.collect(Collectors.toList());
return departmentVoList;
```
使用flatMap操作可以避免使用嵌套的循环,使代码更加简洁易懂。
map.ofentriesf用法
map.ofentriesf 是一个 Java 9 中的新方法,用于创建一个 Map 对象,其键和值由传入的参数决定。它的用法如下:
1. 导入 java.util.Map 和 java.util.stream.Collectors 包。
2. 定义一个 Map 对象,使用 Map.ofEntriesF() 方法创建:
```
import java.util.Map;
import java.util.stream.Collectors;
Map<Integer, String> map = Map.ofEntriesF(
Map.entry(1, "One"),
Map.entry(2, "Two"),
Map.entry(3, "Three")
);
```
上面的代码创建了一个具有三个键值对的 Map 对象,键为整数,值为字符串。
3. 如果需要创建一个具有更多键值对的 Map 对象,可以传入更多的 Map.entry() 参数:
```
Map<Integer, String> map = Map.ofEntriesF(
Map.entry(1, "One"),
Map.entry(2, "Two"),
Map.entry(3, "Three"),
Map.entry(4, "Four"),
Map.entry(5, "Five")
);
```
上面的代码创建了一个具有五个键值对的 Map 对象。
4. 如果需要创建一个具有重复键的 Map 对象,可以使用 Map.entry() 方法创建一个键值对列表,然后将其转换为 Map 对象:
```
import java.util.List;
List<Map.Entry<Integer, String>> entries = List.of(
Map.entry(1, "One"),
Map.entry(2, "Two"),
Map.entry(3, "Three"),
Map.entry(1, "Uno") // 重复键
);
Map<Integer, String> map = entries.stream()
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
System.out.println(map); // {1=Uno, 2=Two, 3=Three}
```
上面的代码创建了一个具有三个键值对的 Map 对象,其中键为 1 的值被覆盖为 "Uno"。
总之,map.ofentriesf 方法可以很方便地创建一个具有指定键值对的 Map 对象。
阅读全文
相关推荐
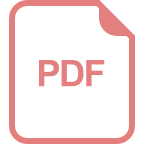
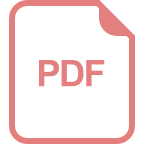
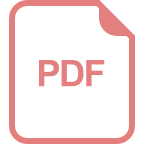
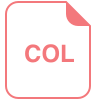
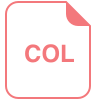











