python确定子类不继承父类的某个方法
时间: 2024-10-25 20:14:57 浏览: 50
在Python中,如果想要防止子类继承父类的特定方法,通常有两种方法:
1. **私有方法**(Private method):通过在方法名前加上两个下划线`__`,可以创建一个只有该类内部能访问的方法,外部包括子类都无法直接调用。例如:
```python
class Parent:
def __private_method(self):
pass
class Child(Parent):
# 子类无法直接调用父类的私有方法
pass
```
2. **不显式地覆盖**(Not overriding the method):如果你不想让子类继承并重写这个方法,你可以不提供其实现。子类会继承到父类的方法签名,但实际上不会做任何事。例如:
```python
class Parent:
def some_method(self):
print("Parent's method")
class Child(Parent):
# 子类没有覆盖 this_method,它将默认调用父类的版本
def some_method(self):
pass
```
在这种情况下,`Child`类的实例调用`some_method`时,会执行的是`Parent`类的`some_method`。
相关问题
Python子类怎么继承父类的构造方法
在Python中,子类继承父类的构造方法(也称为初始化方法或特殊方法`__init__`)是通过直接调用父类的`__init__`函数来实现的。如果你有一个父类`Parent`,并且你想让子类`Child`继承并调用`Parent`的`__init__`,你可以这样做:
```python
class Parent:
def __init__(self, parent_arg):
self.parent_var = parent_arg
class Child(Parent): # 子类继承自父类
def __init__(self, child_arg, *args, **kwargs): # 在子类中,可以添加自己的参数
super().__init__(parent_arg=child_arg) # 调用父类的__init__
self.child_var = child_arg # 添加子类特有的初始化
# 创建子类实例
instance = Child("Child's argument", some_other_arg="value")
```
在这个例子中,`Child`的`__init__`方法中使用了`super().__init__(parent_arg=child_arg)`来调用父类`Parent`的`__init__`,并将传递给它的`child_arg`作为`parent_arg`。这样,当你创建`Child`对象时,会同时初始化父类和子类的属性。
Python面向对象子类继承父类,直接调用父类公共方法的关键词
在 Python 中,如果子类继承父类,子类可以直接调用父类的公共方法,关键词为 `super()`。
例如:
```
class Parent:
def public_method(self):
print("This is a public method of the Parent class.")
class Child(Parent):
def call_parent_method(self):
super().public_method()
```
在这个例子中,`Child` 类继承了 `Parent` 类,它可以直接调用 `Parent` 类的公共方法 `public_method`。你可以这样调用:
```
child = Child()
child.call_parent_method()
```
输出结果为:
```
This is a public method of the Parent class.
```
注意,如果子类重写了父类的方法,那么你可以使用 `super()` 来调用父类的方法。例如:
```
class Parent:
def public_method(self):
print("This is a public method of the Parent class.")
class Child(Parent):
def public_method(self):
print("This is the modified public method of the Child class.")
super().public_method()
```
在这个例子中,`Child` 类重写了 `Parent` 类的 `public_method` 方法。但是,如果你想在子类中调用父类的方法,你可以使用 `super().public_method()` 来调用。
你可以这样调用:
```
child = Child()
child.public_method()
```
输出结果为:
```
This is the modified public method of the Child class.
This is a public method of the Parent class.
```
希望这能帮到你!
阅读全文
相关推荐
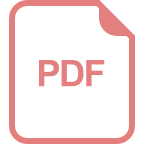
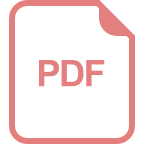
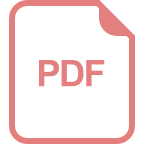













