在C#中使用ADO.NET写一个登录接口:参数为用户名、密码,验证通过,显示true,验证失败,显示false
时间: 2024-09-14 08:08:28 浏览: 35
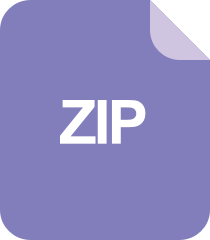
c#WebAPI连接数据库
在C#中,你可以创建一个简单的登录接口来验证用户凭据,通常会涉及到数据库访问。这里我们将使用ADO.NET连接到数据库并检查用户名和密码是否匹配。假设有一个`Users`表,其中包含`Username`和`Password`字段。以下是代码实现:
```csharp
using System;
using System.Data.SqlClient;
public class AuthenticationService
{
private readonly string connectionString;
public AuthenticationService(string connectionString)
{
this.connectionString = connectionString;
}
// 登录方法
public bool AuthenticateUser(string username, string password)
{
bool isValid = false;
try
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string query = "SELECT * FROM Users WHERE Username = @username AND Password = @password;";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@username", username);
command.Parameters.AddWithValue("@password", Encrypt(password)); // 加密密码防止直接存储
SqlDataReader reader = command.ExecuteReader();
if (reader.Read()) // 如果找到一条匹配的数据
isValid = true;
else
Console.WriteLine($"用户名或密码错误:{username}");
reader.Close();
}
}
catch (Exception ex)
{
Console.WriteLine($"数据库操作异常: {ex.Message}");
}
return isValid;
}
private static string Encrypt(string password) => "Encrypt the password here"; // 实际应用中需要加密函数
}
// 示例用法
public static void Main()
{
string connectionString = "your_connection_string_here";
AuthenticationService service = new AuthenticationService(connectionString);
bool isAuthorized = service.AuthenticateUser("example_user", "example_password");
if (isAuthorized)
Console.WriteLine("登录成功");
else
Console.WriteLine("登录失败");
}
```
在这个示例里,`AuthenticateUser`方法接收用户名和密码,通过SQL查询检查是否存在匹配的记录。实际项目中你需要替换`Encrypt`方法为真正的加密算法。
阅读全文
相关推荐
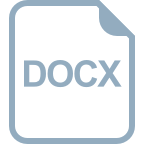
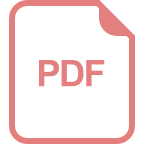
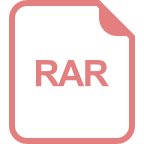
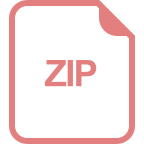
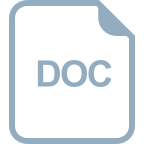
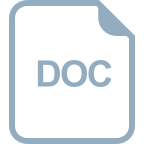
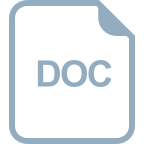
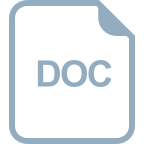
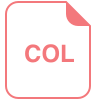
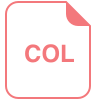
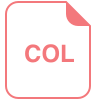
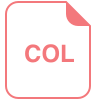
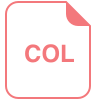
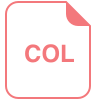
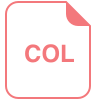
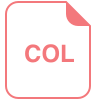
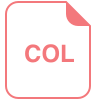
