从n个无序的数中,找出第k小的元素,基于快速排序的思想实现,用C++语言编写
时间: 2024-10-14 08:10:06 浏览: 4
在C++中,可以使用快速排序的思想结合一个大小为k的滑动窗口来解决这个问题。快速排序通常用于对数组进行原地排序,这里我们可以利用其分区过程,即每次将数组分为两部分,一部分所有元素都小于另一部分。在快速排序过程中,我们维护两个指针,左边的`low`指向当前已排序区间的起始位置,右边的`high`指向未排序区间结束位置,然后找到第k小的元素。
以下是基本的C++实现:
```cpp
#include <vector>
using namespace std;
int partition(vector<int>& nums, int low, int high) {
int pivot = nums[high]; // 选择最右侧元素作为基准
int i = (low - 1); // 定义i为小于基准的最后一个元素
for (int j = low; j <= high - 1; j++) {
if (nums[j] < pivot) {
i++; // 把比pivot小的元素移到左边
swap(nums[i], nums[j]);
}
}
swap(nums[i + 1], nums[high]); // 将基准放到正确的位置
return i + 1;
}
int quickSelectKthSmallest(vector<int>& nums, int k, int low, int high) {
if (low == high) { // 如果只有一个元素,那就是kth smallest
return nums[low];
}
int pivotIndex = partition(nums, low, high);
// 当前基准所在的位置就是我们要找的值的位置
if (k == pivotIndex)
return nums[k];
else if (k < pivotIndex)
return quickSelectKthSmallest(nums, k, low, pivotIndex - 1);
else
return quickSelectKthSmallest(nums, k - pivotIndex - 1, pivotIndex + 1, high);
}
int findKthSmallest(vector<int>& nums, int k) {
return quickSelectKthSmallest(nums, k, 0, nums.size() - 1);
}
// 示例
vector<int> arr = {7, 10, 4, 3, 20, 15};
int k = 2;
cout << "第" << k << "小的元素是:" << findKthSmallest(arr, k) << endl;
相关推荐
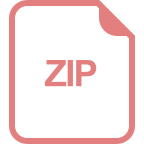
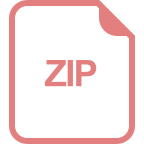
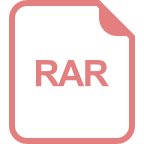














