vue 监听 div 高度
时间: 2024-05-11 10:12:34 浏览: 276
要监听div的高度变化,可以使用Vue的watch和ref属性来实现。具体步骤如下:
1. 在div标签上添加ref属性,例如:ref="myDiv"。
2. 在Vue的data中定义一个变量,例如:divHeight。
3. 在Vue的watch中监听myDiv的高度变化,如果高度发生变化则将新的高度赋值给divHeight变量。
以下是示例代码:
```
<template>
<div ref="myDiv">
// div内容
</div>
</template>
<script>
export default {
data() {
return {
divHeight: 0
}
},
watch: {
'myDiv.offsetHeight'(newVal, oldVal) {
if (newVal !== oldVal) {
this.divHeight = newVal
}
}
}
}
</script>
```
相关问题
vue监听div高度变化
在中监听`div`元素高度变化可以通过`Vue`提供的`$refs`属性以及`Vue`提供的`$watch`函数实现。
首先,在`div`元素上添加一个`ref`属性,例如:
```
<template>
<div ref="example"></div>
</template>
```
然后,在组件中定义一个`computed`属性,用于获取`div`元素的高度:
```
<script>
export default {
computed: {
exampleHeight() {
return this.$refs.example.clientHeight;
}
},
watch: {
exampleHeight(newHeight, oldHeight) {
// 处理高度变化
}
}
}
</script>
```
在`computed`中,使用`this.$refs.example`获取`div`元素,并调用`clientHeight`获取元素的高度。然后在`watch`中监听`exampleHeight`的变化,当高度发生变化时执行相应的处理逻辑。
需要注意的是,由于`$refs`属性是在`mounted`钩子函数中才能获取到,因此需要保证`$refs.example`在`computed`和`watch`中都被正确地引用。同时,需要注意`$refs`属性引用的是原生`DOM`元素,而不是`Vue`组件实例,因此不能在`$refs`上直接调用`Vue`组件实例的方法或属性。
vue 监听 class 高度
Vue 监听 class 高度的一种常见方法是使用 `v-bind` 指令和计算属性。具体步骤如下:
1. 在需要监听高度变化的元素上绑定一个 class,例如 `my-class`。
2. 使用 `v-bind` 指令将这个 class 绑定到一个计算属性上,例如 `myHeight`。
3. 在计算属性中返回这个元素的高度,可以使用 JavaScript 中的 `offsetHeight` 属性获取。
4. 当这个元素的高度发生变化时,计算属性会重新计算并更新视图。
代码示例如下:
```
<template>
<div class="my-class" :class="{ 'my-class-height': myHeight }">
<!-- 元素内容 -->
</div>
</template>
<script>
export default {
data() {
return {
// 其他数据
};
},
computed: {
myHeight() {
return document.querySelector('.my-class').offsetHeight;
},
},
};
</script>
```
在上述示例中,当 `.my-class` 元素的高度发生变化时,`myHeight` 计算属性会重新计算并更新视图。同时,我们还可以通过绑定另一个 class(例如 `my-class-height`)来实现高度变化后的样式更新。注意,在计算属性中访问 DOM 元素时应该避免使用 jQuery 等框架,而是使用原生的 JavaScript 方法。
阅读全文
相关推荐
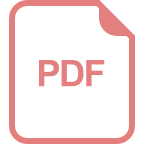
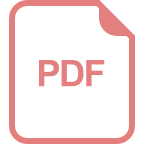
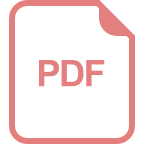













