C++ QT 0.9999小数取前3位显示
时间: 2024-12-15 17:27:38 浏览: 4
在C++中,如果你想要截取QDecimal(QT库中的高精度浮点数类型)的小数部分,并仅保留前三位,你可以使用`setPrecision()`方法设置小数点后的位数,然后通过字符串转换展示。这里是简单的步骤:
```cpp
#include <QDecimal>
#include <QString>
// 创建一个QDecimal对象
QDecimal number = QDecimal::fromString("0.9999");
// 设置小数点后只保留三位
number.setPrecision(3);
// 转换成字符串并显示前3位小数
QString formattedNumber = number.toString();
formattedNumber.truncate(formattedNumber.length() - 4); // 去掉小数点和多余的零
// 输出结果
qDebug() << "Formatted number (truncated to 3 decimal places): " << formattedNumber;
```
在这个例子中,`formattedNumber.truncate()`会去掉字符串末尾多余的零,确保输出总是精确到前三位。
相关问题
C++ QT 0.9999小数取前3位显示不进行保留
在C++中,特别是使用Qt库的时候,如果你遇到想要将0.9999这样的小数取其前三位并精确到特定精度的问题,你可以通过设置`setPrecision()`函数来控制浮点数的显示格式。例如,如果你想只显示前三位,可以这样做:
```cpp
QDoubleSpinBox spinBox; // 假设这是一个QDoubleSpinBox控件
spinBox.setDecimals(3); // 设置最多显示3位小数
spinBox.setFixedPointNotation(true); // 将数值转换为固定小数点表示
spinBox.setValue(0.9999); // 设置值
QString displayValue = spinBox.value().toString('f', 3); // 'f'指定四舍五入规则,3是保留3位
// displayValue现在就是"0.999"(如果超过三位则自动截断)
// 如果你想直接截断而不是四舍五入
displayValue = QString::number(spinBox.value(), 'g', 3).trimmed(); // 'g'表示一般格式,'3'仍保留三位
```
注意这里的`setDecimals(3)`会改变输入框的最大显示位数,而`trimmed()`用于移除多余的零。但是,对于0.9999这种数字,无论怎样设置,结果都会是"0.999",因为它本来就有四位小数。
qt c++ QLabel加载.svg图片
在Qt C++中,QLabel是一个常用的用于显示文本、图像和其他小部件的控件。如果你想在QLabel中加载SVG(Scalable Vector Graphics)图片,你可以通过以下步骤操作:
1. 首先,确保已经包含了`<QtSvg/QSvgRenderer>`库。如果你使用的是Qt5,它应该已经包含在默认安装中;如果用的是Qt6,需要手动添加`QT-svg`模块。
```cpp
#include <QtSvg/QSvgRenderer>
```
2. 创建一个QLabel实例,并设置其大小和布局。
```cpp
QLabel *label = new QLabel(parent);
label->setMinimumSize(200, 200); // 设置合适的大小
```
3. 加载SVG文件,创建一个QSvgRenderer实例,并将渲染结果设置给QLabel。
```cpp
QString svgPath = "path_to_your_svg_file.svg"; // 替换为实际SVG文件路径
QSvgRenderer renderer(svgPath);
// 如果SVG解析成功
if (renderer.isValid()) {
QImage image = renderer.renderedImage();
label->setPixmap(QPixmap::fromImage(image)); // 设置QLabel显示SVG图像
} else {
qDebug() << "Failed to load SVG file.";
}
```
4. 最后,记得连接信号处理函数或者在适当的时候清理QSvgRenderer,避免内存泄漏。
```cpp
connect(&renderer, &QSvgRenderer::finished, []() { delete &renderer; });
```
阅读全文
相关推荐
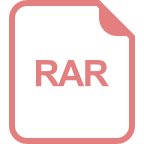













