java多线程异步执行任务
时间: 2024-12-28 09:17:06 浏览: 6
### Java 中实现多线程异步执行任务
#### 使用 `Thread` 类实现异步运行
通过继承 `Thread` 类并覆写其 `run()` 方法来定义线程的任务逻辑。当调用 `start()` 方法时,JVM 将自动调用此方法,在新启动的线程上执行其中的内容。
```java
class MyTask extends Thread {
@Override
public void run() {
System.out.println("MyTask is running on thread " + Thread.currentThread().getName());
// Simulate a time-consuming operation, such as network request or file processing.
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
System.out.println("MyTask has finished.");
}
}
```
创建并启动上述自定义线程对象即可触发对应的异步操作[^1]。
#### 利用 `ExecutorService` 接口简化管理
相比于手动控制生命周期的方式更为推荐的做法是借助于更高层次抽象——即基于池化机制的服务接口 `ExecutorService` 来提交可执行单元给它内部维护着的一组工作进程去完成;这不仅能够有效减少资源消耗还能更好地支持并发场景下的性能优化需求。
```java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class TaskRunner {
private static final ExecutorService executor = Executors.newFixedThreadPool(10);
public static void main(String[] args) throws InterruptedException {
for (int i = 0; i < 10; ++i) {
int taskId = i;
executor.submit(() -> {
System.out.printf("Executing task %d from pool thread %s\n", taskId,
Thread.currentThread().getName());
try {
Thread.sleep((long)(Math.random()*10_000));
} catch (InterruptedException ignored) {}
System.out.printf("Finished executing task %d.\n", taskId);
});
}
// Shutdown the service after all tasks have been submitted.
executor.shutdown();
// Wait until all threads are finish execution or timeout occurs.
while (!executor.isTerminated()) {}
System.out.println("All tasks completed.");
}
}
```
这段程序展示了如何利用固定大小的工作队列来进行批量作业调度,并且可以方便地调整参数以适应不同规模的应用环境要求[^2]。
#### 借助 `Future<V>` 获取返回结果
对于那些希望得到具体计算成果而非仅仅关注过程本身的情况,则可以通过向 `submit(Callable<T> task)` 提交带有产出型签名的目标函数从而获得一个代表未来可能存在的输出凭证实例 —— 即泛型化的 `Future<V>` 对象用于稍后查询实际值是否存在以及尝试读取它们:
```java
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
// Define callable task that returns an integer result upon completion.
static class Summation implements Callable<Integer> {
private final int n;
Summation(int numTerms) { this.n = numTerms; }
@Override
public Integer call() throws Exception {
int sum = 0;
for (int k=1 ;k<=this.n;++k){
sum += k;
}
return sum;
}
}
...
try{
Future<Integer> futureResult = executor.submit(new Summation(100));
doOtherStuff(); // Do other things meanwhile
if(futureResult.isDone()){
System.out.println("Sum of first hundred natural numbers:"+futureResult.get());
}
}catch(Exception ex){
ex.printStackTrace();
}finally{
executor.shutdownNow();
}
```
这里展示了一个简单的累加器作为示范案例说明了怎样结合使用回调模式与延迟求值特性共同作用下达到既保持灵活性又不失效率的目的[^4]。
阅读全文
相关推荐
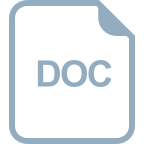
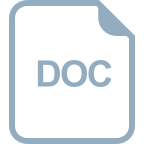
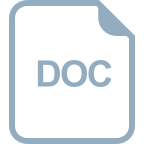
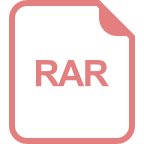
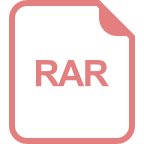
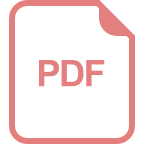
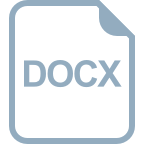
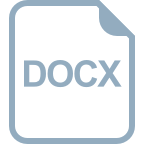
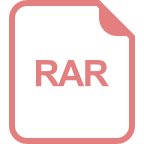
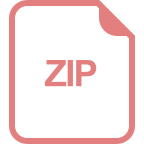
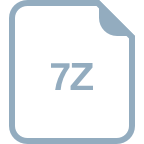
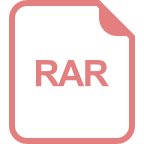
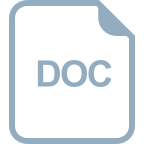
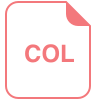

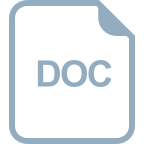
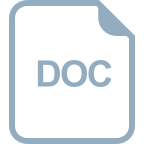
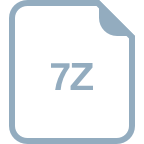
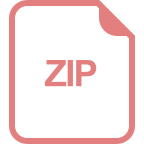
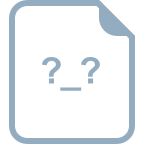