排序,函数可以根据所带参数决定升序或降序
时间: 2024-12-15 14:20:48 浏览: 5
排序是一种数据结构的操作,它按照特定规则将一组元素排列成有序的形式。在编程中,我们通常会定义一种函数,比如`sort()`,这个函数可以接受一个数组或列表作为输入,并允许用户通过传递额外的参数来指定排序的方式,即升序还是降序。
例如,在Python中,我们可以使用`sorted()`函数对列表进行排序,默认情况下是升序排列,如果需要降序排列,可以设置`reverse=True`。类似地,一些通用的排序算法如快速排序、归并排序等也可以通过传入标志或参数来改变排序方向。
```python
# Python 示例
def sort_numbers(numbers, ascending=True):
return sorted(numbers, reverse=not ascending)
numbers = [5, 3, 8, 1, 9]
sorted_ascending = sort_numbers(numbers) # 升序 [1, 3, 5, 8, 9]
sorted_descending = sort_numbers(numbers, False) # 降序 [9, 8, 5, 3, 1]
```
相关问题
对一个整形数序列排序,要求排序函数具有根据参数,决定升序还是降序排序
当你需要对整数序列进行排序,并根据某种条件(升序或降序)来进行,你可以创建一个通用的排序函数,接受一个比较函数作为额外的参数。在C++中,`std::sort`函数就提供了这样的灵活性。以下是一个简单的例子,使用`std::less<int>`(默认情况下按升序排序)和自定义的比较函数`compare_descending`(用于降序排序):
```cpp
#include <algorithm> // 包含sort函数
#include <vector>
// 默认的升序比较函数
bool compare_ascending(int a, int b) {
return a < b;
}
// 降序比较函数
bool compare_descending(int a, int b) {
return a > b; // 返回a是否大于b,即判断是否降序
}
void sort_sequence(std::vector<int>& nums, bool isDescending = false) {
if (isDescending) {
std::sort(nums.begin(), nums.end(), compare_descending);
} else {
std::sort(nums.begin(), nums.end(), compare_ascending); // 使用默认的升序排序
}
}
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 7};
// 升序排序
sort_sequence(numbers);
for (const auto& num : numbers) {
std::cout << num << " ";
}
// 降序排序
sort_sequence(numbers, true);
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
```
在这个例子中,`sort_sequence`函数接受一个整数向量`nums`和一个可选标志`isDescending`,如果设置为`true`,则按照降序排列;否则,默认按升序排列。
argsort函数排序是升序还是降序
argsort函数是NumPy中的一个函数,它的作用是返回数组中元素排序后的索引值。默认情况下,argsort函数返回的是升序排列的索引值。例如,对于一个数组arr=[3, 1, 4, 1, 5, 9, 2, 6, 5, 3],调用argsort函数得到的索引值为[1, 3, 6, 0, 9, 2, 4, 7, 8, 5],这个数组表示将原来的数组升序排列后的索引值。如果要将数组降序排列,可以使用[::-1]反转索引值的顺序,方法如下:
```
import numpy as np
arr = np.array([3, 1, 4, 1, 5, 9, 2, 6, 5, 3])
idx = np.argsort(arr)[::-1]
```
这样就可以得到降序排列的索引值idx=[5, 8, 4, 2, 7, 0, 6, 3, 1, 9],对应的元素值为arr[idx]=[9, 5, 5, 4, 6, 3, 2, 1, 1, 3]。
阅读全文
相关推荐
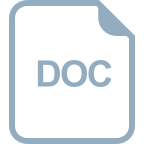
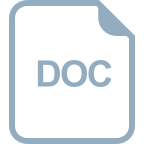
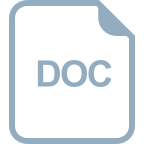
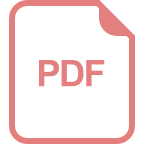
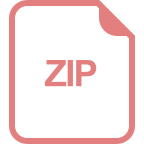
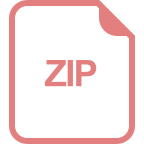










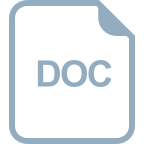