java 以文件流上传
时间: 2023-10-18 19:45:47 浏览: 195
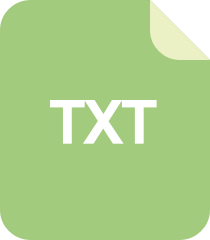
Java以流的形式上传文件

对于 Java 语言来说,可以使用文件流来实现文件上传功能。下面是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUploader {
public static void main(String[] args) throws IOException {
String filePath = "path/to/file.txt";
String uploadUrl = "http://example.com/upload";
File file = new File(filePath);
FileInputStream inputStream = new FileInputStream(file);
URL url = new URL(uploadUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("POST");
// 设置请求头部信息,根据需要设置
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("Content-Disposition", "attachment; filename=\"" + file.getName() + "\"");
OutputStream outputStream = connection.getOutputStream();
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
int responseCode = connection.getResponseCode();
System.out.println("Server response code: " + responseCode);
inputStream.close();
outputStream.close();
}
}
```
在上述代码中,我们通过创建一个 `File` 对象来表示要上传的文件,然后使用 `FileInputStream` 打开输入流。接下来,我们创建一个 `URL` 对象来表示上传的目标 URL,并打开一个 `HttpURLConnection` 连接。
设置连接的一些属性,例如允许输出、请求方法等。然后根据需要设置请求头部信息,包括内容类型和文件名。接着,我们打开输出流,并将文件内容写入输出流中。
最后,我们可以通过调用 `getResponseCode()` 方法获取服务器的响应代码来判断上传是否成功。
请注意,在实际使用中,你需要将 `filePath` 和 `uploadUrl` 替换为你自己的文件路径和上传 URL。
阅读全文
相关推荐
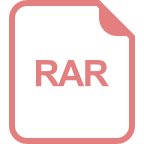
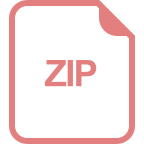
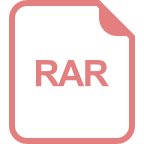
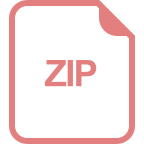
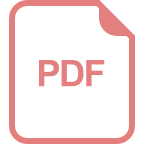
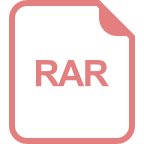
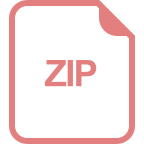
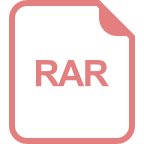
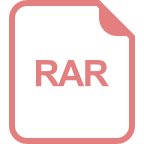
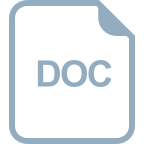
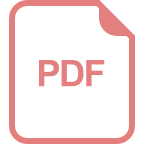
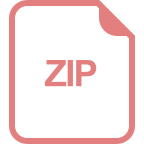