Sqlite3 python some code
时间: 2024-12-22 08:11:10 浏览: 10
要使用SQLite3在Python中存储数据,首先确保已连接到数据库(这里我们创建了一个内存数据库)[^1]:
```python
import sqlite3
# 连接到SQLite数据库 (如果尚未存在,则会自动创建)
conn = sqlite3.connect(":memory:", check_same_thread=False)
# 创建一个游标对象,用于执行SQL命令
cursor = conn.cursor()
# 假设你有数据(比如从网络获取的数据)
data_to_store = {
'key1': 'value1',
'key2': 'value2'
}
# 插入数据到一个表(假设有一个名为`my_table`的表)
table_name = "my_table"
cursor.execute(f"CREATE TABLE IF NOT EXISTS {table_name} (key TEXT PRIMARY KEY, value TEXT)")
# 执行插入操作
for key, value in data_to_store.items():
cursor.execute(f"INSERT INTO {table_name} (key, value) VALUES (?, ?)", (key, value))
# 提交事务以保存更改
conn.commit()
```
如果你遇到与线程同步的问题,可能是因为`check_same_thread=False`参数允许你在多线程环境中非阻塞地访问数据库。确保关闭数据库连接时,在线程结束之前完成:
```python
def worker_function(data):
with conn:
... # 执行上述代码,处理数据并写入数据库
# 在你的线程循环中调用worker_function
from threading import Thread
threads = []
for _ in range(10): # 示例,创建10个线程
thread = Thread(target=worker_function, args=(data,))
threads.append(thread)
thread.start()
# 等待所有线程完成
for thread in threads:
thread.join()
```
阅读全文
相关推荐




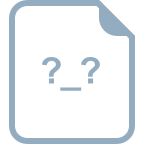












