用c语言编写七桥问题
时间: 2024-11-05 22:26:37 浏览: 0
七桥问题是一个经典的算法设计题目,它涉及路径寻找,通常通过回溯法或深度优先搜索(DFS)来解决。以下是用C语言编写基本的七桥问题求解思路:
```c
#include <stdio.h>
#include <stdlib.h>
#define N 7 // 代表七座桥
int matrix[N][N] = {
{0, 1, 0, 0, 0, 0, 1},
{1, 0, 1, 0, 0, 1, 0},
{0, 1, 0, 1, 0, 0, 0},
{0, 0, 1, 0, 1, 0, 0},
{0, 0, 0, 1, 0, 1, 0},
{0, 1, 0, 0, 1, 0, 1},
{1, 0, 0, 0, 0, 1, 0} // 可能有其他排列,这里是个例子
};
// 检查从起点到终点是否合法
int isValid(int i, int j) {
return (i >= 0 && i < N && j >= 0 && j < N && matrix[i][j] == 0);
}
// 回溯函数,解决八皇后问题
void solve(int row, int *solution) {
if (row == N) {
for (int i = 0; i < N; ++i)
printf("%d ", solution[i]);
printf("\n");
return;
}
for (int col = 0; col < N; ++col) {
if (isValid(row, col)) {
solution[row] = col;
solve(row + 1, solution); // 继续往下一个行填充
}
}
}
int main() {
int solution[N];
solve(0, solution);
return 0;
}
阅读全文
相关推荐
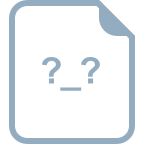
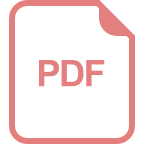
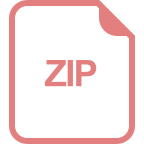

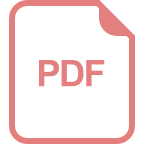
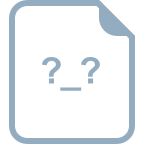
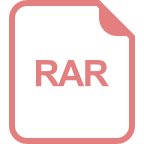
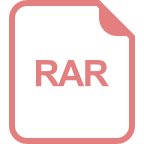
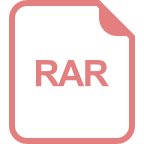
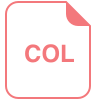

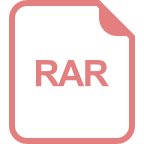
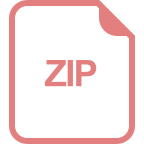
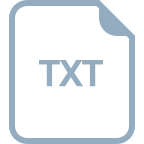
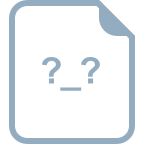
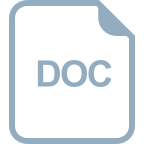

