基于链表用c语言完成一个简易的图书管理系统
时间: 2024-09-19 20:01:37 浏览: 42
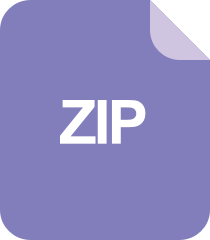
基于链表的图书管理系统项目
基于链表实现一个简单的图书管理系统,我们可以设计一个包含书籍信息的数据结构,并使用单向链表存储每本书。这里是一个简化的示例:
首先,我们创建一个`Book`结构体,包含书名、作者和ID等基本属性。
```c
typedef struct Book {
char title[50];
char author[50];
int id;
struct Book* next; // 链接指针
} Book;
```
接下来,我们需要创建链表相关的函数:
1. **初始化链表**:创建一个空链表
```c
Book* createEmptyList() {
Book* head = NULL;
return head;
}
```
2. **添加新书**:在链表末尾插入新的`Book`节点
```c
void addBook(Book* list, const char* title, const char* author, int id) {
Book* newNode = (Book*)malloc(sizeof(Book));
if (newNode != NULL) {
strcpy(newNode->title, title);
strcpy(newNode->author, author);
newNode->id = id;
newNode->next = NULL;
if (list == NULL) {
list = newNode;
} else {
Book* temp = list;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
}
```
3. **查找书籍**:遍历链表寻找特定ID的书籍
```c
Book* searchBook(Book* list, int id) {
Book* temp = list;
while (temp != NULL) {
if (temp->id == id) {
return temp;
}
temp = temp->next;
}
return NULL; // 如果未找到,返回NULL
}
```
4. **删除书籍**:根据ID从链表中移除指定的书
```c
void deleteBook(Book* list, int id) {
if (list == NULL) {
return;
}
if (list->id == id) {
Book* temp = list;
list = list->next;
free(temp);
return;
}
Book* prev = list;
Book* curr = list->next;
while (curr != NULL && curr->id != id) {
prev = curr;
curr = curr->next;
}
if (curr != NULL) {
prev->next = curr->next;
free(curr);
}
}
```
5. **打印所有书籍**:用于展示链表中的书籍信息
```c
void displayBooks(Book* list) {
Book* temp = list;
while (temp != NULL) {
printf("ID: %d, Title: %s, Author: %s\n", temp->id, temp->title, temp->author);
temp = temp->next;
}
}
```
阅读全文
相关推荐
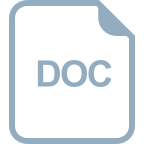
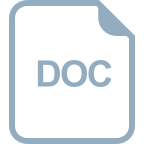
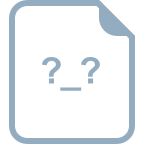
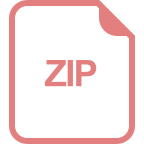
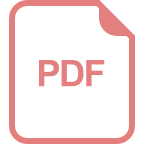
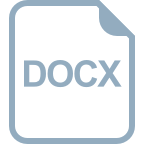
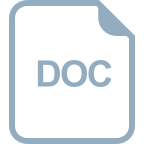
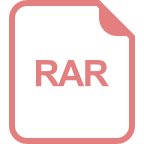
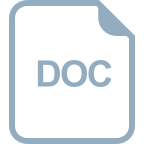
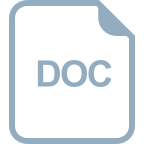


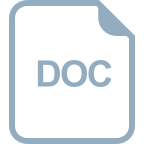
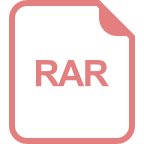
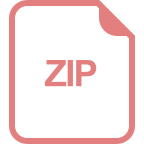
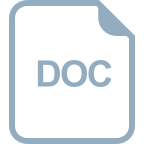
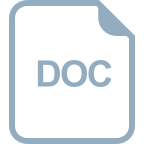
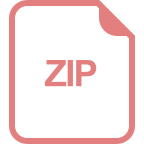