C语言,计算单链表中元素个数
时间: 2024-10-15 12:18:28 浏览: 31
在C语言中,计算单链表中元素个数通常涉及到遍历链表的过程。单链表是由节点构成的数据结构,每个节点包含两个部分:数据域和指向下一个节点的指针。以下是基本步骤:
1. 定义一个函数`count_elements`,它接收链表的头结点作为参数。
2. 初始化一个变量`count`为0,用于存储链表中的元素个数。
3. 使用一个while循环,条件通常是当前节点不为空(`node != NULL`):
a. `count++`: 每次进入循环,`count`自增1表示找到了一个元素。
b. 更新节点指针`node = node->next`,移动到下一个节点。
4. 循环结束后,`count`即为链表中元素的数量。
示例代码片段如下:
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
int count_elements(Node* head) {
int count = 0;
while (head != NULL) {
count++;
head = head->next;
}
return count;
}
// ... 其他链表操作函数
int main() {
// 创建链表并设置头结点
Node* list = create_list(); // 假设create_list()是一个已有的创建链表函数
int length = count_elements(list);
printf("链表中有 %d 个元素.\n", length);
return 0;
}
```
阅读全文
相关推荐
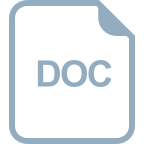
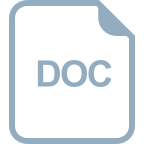
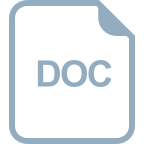

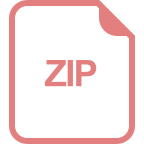
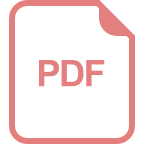
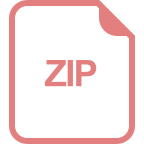
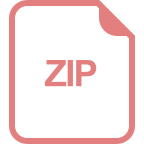
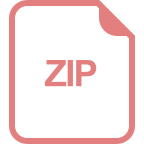
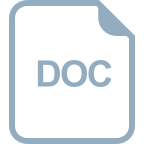
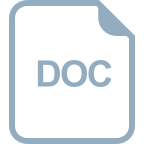
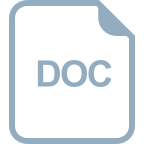
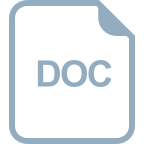
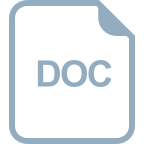
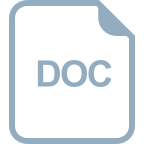
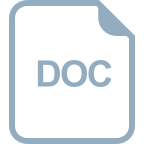
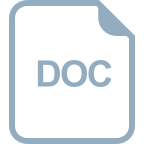
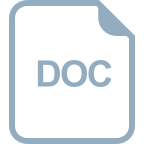
