使用c语言、根据输入的日期,计算是这一年的第几天。输入保证年份为4位数且日期合法。 输入描述:输入一行,每行空格分割,分别是年,月,日。 输出描述:输出是这一年的第几天
时间: 2024-12-13 11:21:03 浏览: 7
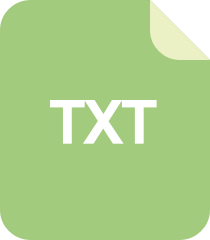
根据日期计算星期几的C语言代码
在C语言中,要根据输入的日期计算这一年中的天数,可以利用闰年的规则(闰年2月有29天)和月份天数的不同。这里我们可以编写一个简单的函数来实现这个功能。以下是一个可能的实现步骤:
1. **判断是否是闰年**:
- 如果年份能被4整除但不是100的倍数,或者能被400整除,则为闰年。
2. **确定每个月的天数**:
- 1月和12月固定为31天。
- 2月在非闰年为28天,在闰年为29天。
- 其他各月分别为30天。
3. **逐月累加天数**:
- 从1月开始,每月加上对应的天数,直到给定的月份。
4. **处理输入**:
- 读取用户输入的年、月、日,验证其合法性。
5. **计算并输出结果**:
- 调用上述函数后,输出该日期是一年的第多少天。
下面是一个简化版的代码示例:
```c
#include <stdio.h>
int days_in_month(int month, int year) {
switch(month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
return is_leap_year(year) ? 29 : 28; // 判断是否为闰年
}
}
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int main() {
int year, month, day;
scanf("%d %d %d", &year, &month, &day);
if (!is_valid_date(year, month, day)) { // 自定义函数判断输入是否合法
printf("Invalid date.\n");
} else {
int total_days = days_in_month(month, year) + (month > 1 ? days_in_month(month - 1, year) : 0) + day;
printf("The date is the %dth day of %d.\n", total_days, year);
}
return 0;
}
```
注意:此代码示例假设了用户输入的月份始终在1到12之间,并没有处理非法输入。在实际项目中,还需要添加更多的错误检查和输入处理部分。对于输入验证部分,你需要自行编写函数,例如`is_valid_date()`,检查输入的年月日是否有效。
阅读全文
相关推荐
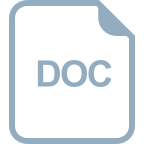
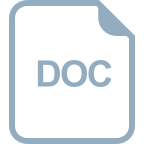
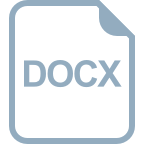
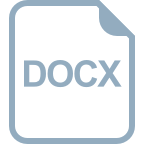
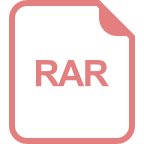
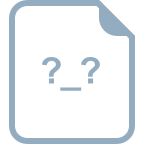
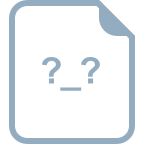
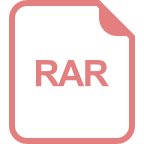
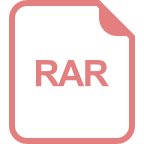
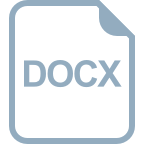
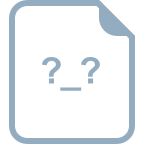
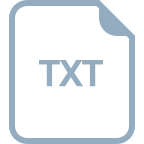
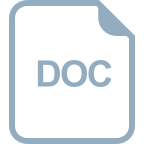