pyqt5 制作12306
时间: 2024-01-20 19:00:38 浏览: 25
12306是一个广泛使用的火车票预订系统,为了制作一个类似的系统,可以使用PyQt5这个强大的Python框架来实现。首先,可以利用PyQt5的各种组件,如按钮、文本框和表格等来设计系统的用户界面,使用户可以方便地进行车票的查询、预订和支付等操作。其次,可以利用PyQt5提供的信号与槽机制来实现各个组件之间的交互,比如当用户点击查询按钮时,系统会根据输入的信息从数据库中查询相应的车次信息并显示在表格中。另外,PyQt5还可以帮助系统实现数据的存储和处理,比如用户的个人信息和订单信息可以通过PyQt5与数据库进行交互并进行持久化存储。此外,PyQt5还可以实现系统的多线程功能,当多个用户同时访问系统时,可以利用PyQt5实现并发处理,提高系统的并发能力。最后,利用PyQt5的图形绘制功能,可以为系统添加各种图形化的元素,如地图、座位图等,使用户能够清晰直观地了解车次的相关信息。综上所述,借助PyQt5这个强大的工具,可以高效地制作一个功能完善、界面友好的12306火车票预订系统。
相关问题
pyqt5制作视频播放器
PyQt5是一个功能强大的Python GUI工具包,它可以用来制作各种类型的应用程序,包括视频播放器。制作一个简单的视频播放器可以通过以下步骤来实现。
首先,我们需要安装PyQt5和相应的多媒体模块。然后,我们可以使用Qt Designer来设计视频播放器的界面,包括播放按钮、暂停按钮、进度条等控件。我们可以使用信号和槽来连接这些控件,使得它们能够与视频文件进行交互。
接下来,我们需要使用QMediaPlayer类来实现视频的播放功能。我们可以通过QFileDialog来选择要播放的视频文件,然后将其加载到QMediaPlayer中。我们还可以使用QVideoWidget来显示视频画面,并将其放置在播放器界面中。
在处理视频播放过程中,我们可以通过QMediaPlayer的信号来实时更新进度条和显示视频的播放状态。我们还可以通过QMediaPlayer的方法来控制视频的播放、暂停、停止等操作。
最后,我们可以将所有的代码组织起来,构建一个完整的视频播放器应用程序。我们可以通过PyQt5的打包工具来将这个应用程序打包成可执行文件,这样用户就可以在不同的操作系统上运行它了。
总的来说,使用PyQt5制作视频播放器可以让我们方便地创建一个功能完备的视频播放应用程序,并且可以充分发挥Python和Qt的优势,实现更多自定义的需求和功能。
pyqt5制作首页框架
首先,你需要安装PyQt5库。可以使用以下命令来安装:
```
pip install PyQt5
```
然后,你可以创建一个基本的PyQt5应用程序框架,如下所示:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QGridLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('首页')
self.setGeometry(100, 100, 800, 600)
central_widget = QWidget()
self.setCentralWidget(central_widget)
grid_layout = QGridLayout()
central_widget.setLayout(grid_layout)
label = QLabel('欢迎来到首页!')
grid_layout.addWidget(label, 0, 0)
if __name__ == '__main__':
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
```
这个示例创建了一个简单的主窗口,并在窗口中添加了一个标签。你可以根据自己的需要添加其他控件和布局。
相关推荐
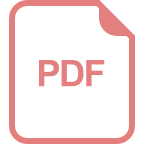
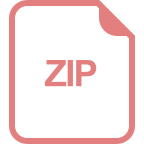
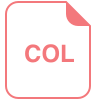
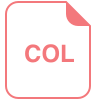
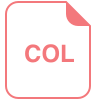









