mybatisplus自定义ID生成策略
时间: 2023-11-29 09:47:27 浏览: 212
在MybatisPlus中,我们可以通过自定义ID生成器来实现自定义ID生成策略。以下是两种自定义ID生成器的方式:
1. 使用配置类
```java
@Bean
public IdentifierGenerator idGenerator() {
return new CustomIdGenerator();
}
```
2. 通过MybatisPlusPropertiesCustomizer自定义
```java
@Bean
public MybatisPlusPropertiesCustomizer plusPropertiesCustomizer() {
return plusProperties -> plusProperties.getGlobalConfig().setIdentifierGenerator(new CustomIdGenerator());
}
```
其中,CustomIdGenerator是我们自定义的ID生成器类,需要实现IdentifierGenerator接口,并重写generate方法来生成自定义的ID。
相关问题
mybatisplus自定义主键生成策略
mybatisplus支持自定义主键生成策略,需要实现IdentifierGenerator接口。具体步骤如下:
1. 实现IdentifierGenerator接口,重写generate方法,生成自定义主键。
```java
public class CustomIdGenerator implements IdentifierGenerator {
@Override
public Serializable generate(SharedSessionContractImplementor session, Object object) throws HibernateException {
// 生成自定义主键
return "custom_id_" + UUID.randomUUID().toString();
}
}
```
2. 在实体类中使用@TableId注解指定主键生成策略为自定义。
```java
public class User {
@TableId(type = IdType.ASSIGN_ID, generator = "customIdGenerator")
private String id;
// ...
}
```
3. 在MybatisPlus配置类中注册自定义主键生成策略。
```java
@Configuration
public class MybatisPlusConfig {
@Bean
public IdentifierGenerator customIdGenerator() {
return new CustomIdGenerator();
}
}
```
mybatisplus 自定义主键生成器
在 MyBatis Plus 中,你可以自定义主键生成器来生成自定义的主键值。以下是一个示例:
1. 创建一个实现了 IdentifierGenerator 接口的自定义主键生成器类,例如 CustomIdGenerator:
```java
public class CustomIdGenerator implements IdentifierGenerator {
@Override
public Serializable nextId(Object entity) {
// 这里编写自己的主键生成逻辑
// 返回生成的主键值
}
}
```
2. 在实体类中使用 @TableId 注解指定使用自定义主键生成器,例如:
```java
public class User {
@TableId(type = IdType.ASSIGN_ID, generator = "customIdGenerator")
private Long id;
// 其他属性和方法省略
}
```
3. 在 MyBatis Plus 的配置文件中配置自定义主键生成器,例如 application.properties 或 application.yml:
```yaml
mybatis-plus:
global-config:
db-config:
id-type: auto # 设置全局的主键类型为自动增长
configuration:
id-generator-type: custom # 设置自定义主键生成器
```
4. 在 MyBatis Plus 的配置类中将自定义主键生成器注册到 MyBatis 的全局配置中,例如:
```java
@Configuration
public class MybatisPlusConfig {
@Bean
public IdentifierGenerator customIdGenerator() {
return new CustomIdGenerator();
}
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
// 添加其他拦截器
return interceptor;
}
}
```
通过以上步骤,你就可以使用自定义的主键生成器来生成自定义的主键值了。请根据你的实际需求来编写自己的主键生成逻辑。
阅读全文
相关推荐
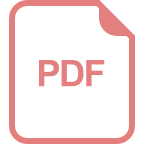


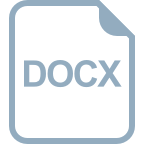
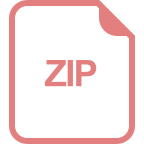






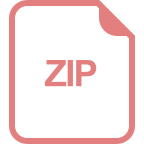


