fastapi 构建一个登陆界面,并按照用户角色跳转不同页面,并用session记录管理账号登陆状态,包括对应的html文件
时间: 2024-09-11 21:05:02 浏览: 15
FastAPI 是一个现代、快速(高性能)的 Web 框架,用于构建 API。使用 FastAPI,你可以构建一个简单的登录界面,并根据用户的角色重定向到不同的页面。以下是一个简单的示例,展示如何使用 FastAPI 和 FastAPI 的内置功能,如 Session 和 OAuth2PasswordBearer,来实现这一功能。
首先,你需要安装 FastAPI 和 Uvicorn 服务器(如果你还没有安装的话):
```bash
pip install fastapi uvicorn
```
接下来,创建一个简单的 FastAPI 应用程序:
```python
from fastapi import FastAPI, Depends, HTTPException, status, Session
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from fastapi.responses import HTMLResponse
from fastapi.templating import Jinja2Templates
from typing import Optional
app = FastAPI()
# 假设的用户数据库
# 实际应用中,应该使用数据库来存储和查询用户信息
users_db = {
"user1": {
"username": "user1",
"password": "password1",
"role": "admin"
},
"user2": {
"username": "user2",
"password": "password2",
"role": "user"
}
}
# 模拟 session 管理器
# 实际应用中应该使用安全的方式存储 session,例如使用加密 cookie 或第三方 session 管理
SECRET_KEY = "mysecretkey"
# OAuth2 密码 bearer 实例
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
templates = Jinja2Templates(directory="templates")
@app.post("/token")
async def login_for_access_token(form_data: OAuth2PasswordRequestForm = Depends()):
user = authenticate_user(form_data.username, form_data.password)
if not user:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Incorrect username or password",
headers={"WWW-Authenticate": "Bearer"},
)
session = Session() # 这里仅作为示例,实际使用时应该创建一个真正的 session
session.update(user)
return {"access_token": session, "token_type": "bearer"}
def authenticate_user(username: str, password: str):
user = users_db.get(username)
if not user or user["password"] != password:
return False
return user
@app.get("/")
async def read_main(session: Session = Depends(oauth2_scheme)):
if "username" not in session:
return RedirectResponse("/token")
user_role = session.get("role")
if user_role == "admin":
return RedirectResponse(url="/admin")
else:
return RedirectResponse(url="/user")
@app.get("/admin", response_class=HTMLResponse)
async def read_admin(session: Session = Depends(oauth2_scheme)):
if session.get("role") != "admin":
raise HTTPException(status_code=403, detail="Not authorized to access this page")
return templates.TemplateResponse("admin.html", {"request": None})
@app.get("/user", response_class=HTMLResponse)
async def read_user(session: Session = Depends(oauth2_scheme)):
if session.get("role") != "user":
raise HTTPException(status_code=403, detail="Not authorized to access this page")
return templates.TemplateResponse("user.html", {"request": None})
# html模板
# templates/admin.html
# <html>
# <head>
# <title>Admin Page</title>
# </head>
# <body>
# <h1>Welcome to the admin page!</h1>
# </body>
# </html>
# templates/user.html
# <html>
# <head>
# <title>User Page</title>
# </head>
# <body>
# <h1>Welcome to the user page!</h1>
# </body>
# </html>
```
在这个例子中,我们定义了两个 HTML 页面:`admin.html` 和 `user.html`。这些文件应该放在与你的 Python 脚本同一目录下的 `templates` 文件夹中。
这里的关键步骤是:
1. 创建一个 OAuth2PasswordBearer 实例来处理登录请求。
2. 创建一个登录函数 `/token` 来验证用户凭据,并返回一个临时的 session(在这个示例中仅用于说明,实际应用中不应该这样使用)。
3. 通过装饰器 `Depends` 来获取 session 信息,并根据用户角色重定向到不同的页面。
4. 创建两个路由 `/admin` 和 `/user` 来返回对应的 HTML 页面。
请注意,这个示例仅用于演示目的,实际部署时应该使用安全的用户认证和 session 管理机制。例如,可以使用数据库来存储用户信息,使用 JWT(JSON Web Tokens)进行安全的 token 生成和验证。
相关推荐
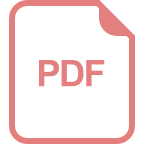
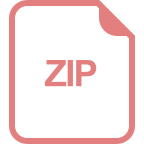
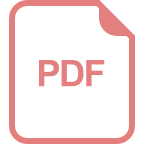














