springboot mybatis 执行sql文件
时间: 2024-01-31 19:10:40 浏览: 34
在SpringBoot中,可以使用MyBatis的MapperScannerConfigurer来执行SQL文件。下面是一个示例配置:
1. 在pom.xml文件中添加MyBatis和MySQL的依赖:
```xml
<dependencies>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
</dependencies>
```
2. 创建一个SQL脚本文件,例如`init.sql`,并将需要执行的SQL语句写入该文件。
3. 在`application.properties`或`application.yml`配置文件中添加以下配置:
```properties
# MyBatis
mybatis.mapper-locations=classpath:mapper/*.xml
# SQL脚本
spring.datasource.schema=classpath:init.sql
spring.datasource.initialization-mode=always
```
或者
```yaml
# MyBatis
mybatis:
mapper-locations: classpath:mapper/*.xml
# SQL脚本
spring:
datasource:
schema: classpath:init.sql
initialization-mode: always
```
4. 创建一个实现了`ApplicationRunner`接口的类,例如`SqlRunner`,并在`run`方法中执行SQL脚本:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.stream.Collectors;
@Component
public class SqlRunner implements ApplicationRunner {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void run(ApplicationArguments args) throws Exception {
executeSqlScript("init.sql");
}
private void executeSqlScript(String scriptPath) throws IOException {
PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
Resource[] resources = resolver.getResources("classpath:" + scriptPath);
for (Resource resource : resources) {
String sql = new BufferedReader(new InputStreamReader(resource.getInputStream()))
.lines().collect(Collectors.joining("\n"));
jdbcTemplate.execute(sql);
}
}
}
```
这样,在SpringBoot启动时,会自动执行`init.sql`中的SQL语句。
相关推荐
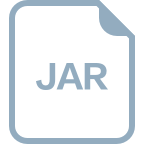
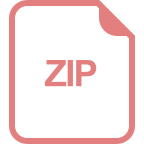
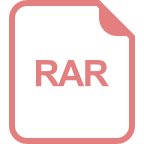














