Selenium如何处理滚动过程中的异步加载?
时间: 2024-09-26 09:10:48 浏览: 30
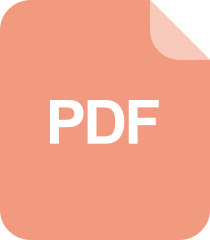
Python使用Selenium爬取淘宝异步加载的数据方法

在Selenium滚动过程中处理异步加载的内容,我们需要结合一些额外的技术来确保所有内容都已加载完毕。因为异步加载通常是基于JavaScript运行的,所以我们可以利用`WebDriverWait`和`expected_conditions`提供的条件来等待元素变得可见或者某些特定状态变化。
以下是一个处理滚动并等待新内容加载完整的示例:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.action_chains import ActionChains
def scroll_and_wait(driver, scroll_element, wait_time):
action = ActionChains(driver)
while True:
action.move_to_element(scroll_element).perform()
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
try:
WebDriverWait(driver, wait_time).until(
EC.visibility_of_element_located((By.XPATH, 'xpath_to_your_visible_element')) or \
EC.presence_of_all_elements_located((By.XPATH, 'xpath_to_newly_loaded_content'))
)
print("All new content loaded.")
break
except TimeoutException:
pass # 如果超时还未加载,继续下一轮滚动
# 使用方法
driver = webdriver.Chrome()
scroll_element = driver.find_element(By.ID, "your-scroll-element")
scroll_and_wait(driver, scroll_element, 5) # 设置滚动等待时间
```
在这个例子中,我们首先移动鼠标到滚动区域,然后滚动到底部。`WebDriverWait`会等待指定的元素变得可见(`EC.visibility_of_element_located`)或出现新的内容(`EC.presence_of_all_elements_located`)。如果在这段时间内没有达到预期状态,就会再次滚动并尝试。
阅读全文
相关推荐
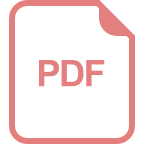
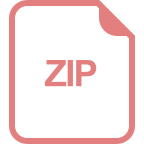

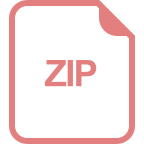
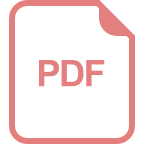
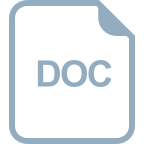
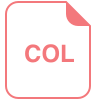
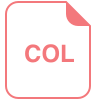
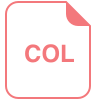
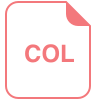
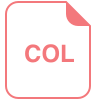
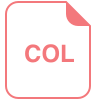
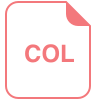
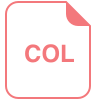




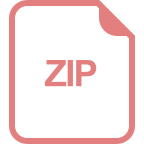