webclient下载
时间: 2023-11-08 07:06:05 浏览: 166
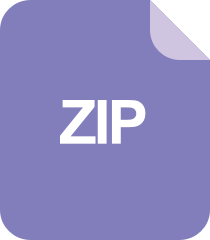
WebClient下载文件

WebClient 是 .NET Framework 中的一个类,用于在客户端应用程序中下载文件或数据。使用 WebClient 可以轻松地从 Internet 上下载文件,也可以将数据上传到 Web 服务器。
以下是使用 WebClient 下载文件的示例代码:
```csharp
using System.Net;
class Program
{
static void Main(string[] args)
{
using (WebClient client = new WebClient())
{
client.DownloadFile("http://example.com/file.txt", "file.txt");
}
}
}
```
这个示例代码会从 http://example.com/file.txt 下载文件,并将其保存为 file.txt。
阅读全文
相关推荐
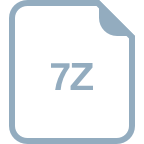
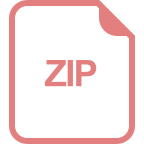
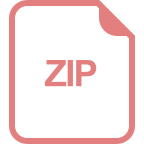
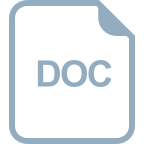
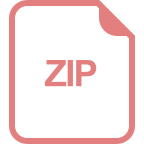
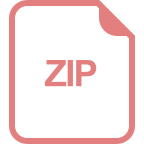
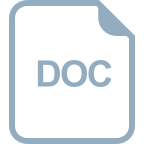
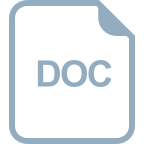


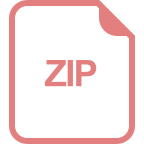
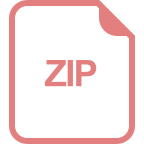
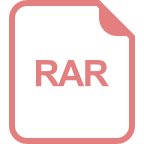
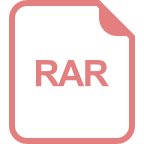
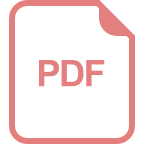
