编写一个学生类,要求该类完成如下功能:(1)输出每个学生的姓名、学号、成绩;(2)统计并输出学生的总人数、总成绩、平均成绩、最高成绩、最低成绩。c++编写
时间: 2024-10-11 16:10:32 浏览: 19
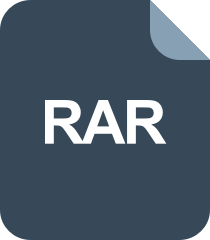
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

在C++中,我们可以创建一个名为`Student`的类来实现这个需求。下面是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 包含sort函数
class Student {
public:
std::string name;
int id;
double score;
// 构造函数
Student(const std::string& n, int i, double s) : name(n), id(i), score(s) {}
// 输出学生信息
void display() {
std::cout << "姓名:" << name << ", 学号:" << id << ", 成绩:" << score << std::endl;
}
};
// 定义全局变量用于存储数据
std::vector<Student> students; // 用于存放所有学生
double total_score = 0.0, max_score = 0.0, min_score = INFINITY; // 总分、最高分和最低分初始值
// 添加新学生到学生列表
void addStudent(const std::string& n, int i, double s) {
students.push_back(Student(n, i, s));
total_score += s;
if (s > max_score)
max_score = s;
else if (s < min_score)
min_score = s;
}
// 统计并输出结果
void printSummary() {
std::cout << "总人数:" << students.size() << std::endl;
std::cout << "总成绩:" << total_score << ", 平均成绩:" << total_score / students.size() << std::endl;
std::cout << "最高成绩:" << max_score << ", 最低成绩:" << min_score << stdendl;
}
int main() {
// 示例添加学生
addStudent("张三", 1001, 95);
addStudent("李四", 1002, 88);
addStudent("王五", 1003, 99);
// 显示单个学生信息
Student* student1 = &students[0];
student1->display();
// 打印所有学生的总结信息
printSummary();
return 0;
}
```
在这个例子中,我们首先定义了一个`Student`类,包含姓名、学号和成绩属性。接着,我们定义了添加学生和打印总结的功能。在`main`函数中,我们创建了几名学生并展示了如何操作他们。
阅读全文
相关推荐
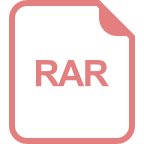
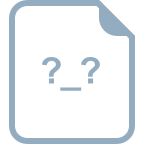












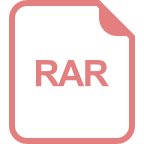
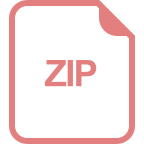
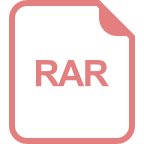