能否提供一个仅用Java编写的自定义哈希表(Hash List)实现的示例代码?
时间: 2024-11-27 14:10:49 浏览: 21
当然可以。在Java中,我们可以使用HashMap来创建一个自定义的哈希表,因为HashMap是一个内置的数据结构,它实现了Map接口,提供了键值对的存储和查找功能。以下是一个简单的自定义哈希表(类似HashMap的简化版)实现示例:
```java
import java.util.HashMap;
class CustomHashTable<K, V> {
private HashMap<K, V> map; // 使用HashMap作为底层存储
public CustomHashTable() {
this.map = new HashMap<>();
}
// 插入元素
public void put(K key, V value) {
map.put(key, value);
}
// 获取元素
public V get(K key) {
return map.get(key);
}
// 删除元素
public void remove(K key) {
map.remove(key);
}
// 判断元素是否存在
public boolean containsKey(K key) {
return map.containsKey(key);
}
}
public class Main {
public static void main(String[] args) {
CustomHashTable<String, Integer> customTable = new CustomHashTable<>();
customTable.put("One", 1);
customTable.put("Two", 2);
System.out.println(customTable.get("One")); // 输出:1
if (customTable.containsKey("Three")) {
System.out.println("Key exists!"); // 输出:Key exists!
} else {
System.out.println("Key not found!");
}
customTable.remove("One");
System.out.println(customTable.containsKey("One")); // 输出:false
}
}
```
这个例子中,`CustomHashTable`类就是一个自定义的哈希表,它包含了插入、获取、删除和检查键存在性的基本操作。请注意,这只是一个简化的版本,实际应用中可能需要添加更多的方法,如容量调整、冲突解决等。
阅读全文
相关推荐
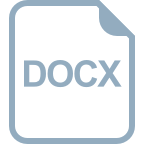
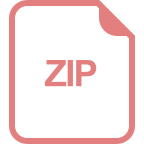
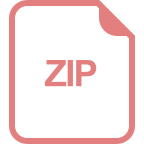
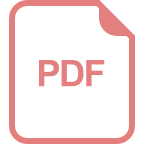
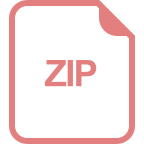