pygame输出文本框
时间: 2024-12-28 20:21:12 浏览: 9
### 创建和显示 Pygame 文本框
为了在 Pygame 中创建并显示文本框,可以基于所提供的信息构建一个较为完整的解决方案。下面是一个综合性的方法来实现这一目标。
#### 初始化 Pygame 和设置环境变量
首先初始化 Pygame 并配置必要的环境变量以便支持多语言输入:
```python
import pygame
import os
os.environ['SDL_IME_SHOW_UI'] = "1"
pygame.init()
screen = pygame.display.set_mode((640, 640))
font = pygame.font.Font(None, 32)
clock = pygame.time.Clock()
input_box = pygame.Rect(100, 100, 140, 32)
color_inactive = pygame.Color('lightskyblue3')
color_active = pygame.Color('dodgerblue2')
color = color_inactive
active = False
text = ''
done = False
```
这段代码设置了屏幕尺寸、字体大小以及定义了一个矩形作为文本框的位置[^2]。
#### 处理事件循环中的用户交互
接下来处理用户的键盘输入和其他动作,比如点击文本框使其激活或失去焦点:
```python
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.MOUSEBUTTONDOWN:
# 如果鼠标点击位置位于文本框内,则切换活动状态;否则关闭它。
if input_box.collidepoint(event.pos):
active = not active
else:
active = False
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
print(text)
text = '' # 清空文本框内容
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((30, 30, 30))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
clock.tick(30)
```
此部分实现了当文本框被选中时改变颜色,并允许通过 `K_RETURN` 键提交输入的内容或将新字符追加到现有字符串之后的功能。
#### 支持自动换行功能
为了让文本框能够适应更多文字而不溢出边界,还需要加入自动换行逻辑。这可以通过检测当前行宽度是否超过了设定的最大宽度来进行调整。不过上述基础版本已经展示了基本原理,更复杂的特性可以根据需求进一步开发。
阅读全文
相关推荐

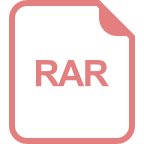





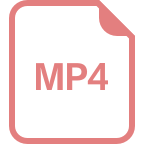

